In this article, we will use the Beckhoff TF6421 to read an XML File and transfer the result to a PLC variable. Let’s get started!
Reference Video
Beckhoff.Let’s read data from XML File via TF6421
TF6421?
The TwinCAT XML Server TF6421 provides a simple PLC library for writing to and reading from XML files; the XML Server also enables implementations such as loading initialisation data, which is often required when starting up a machine, for example.
PLC variables can also be formatted and stored in XML files containing PLC comments; the variable structure of the XML document matches the structure of the PLC variables.
System Requirment
Structure
This is the structure of the TF6421 XML Server.
TwinCAT XML Server
The TwinCAT XML Server is a service that starts/stops together with TwinCAT and is the link between TwinCAT and the XML file.
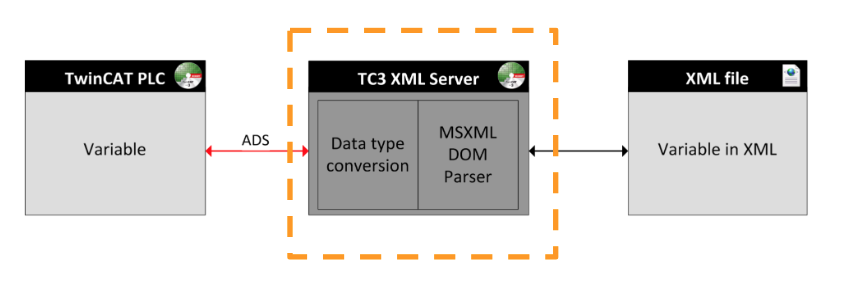
PLC Library
PLC library The PLC library has four Function Blocks, which allow data to be written from the PLC library to an XML file or read from an XML file. Variables can be formatted and saved in an XML file and variables can be initialised in TwinCAT from the XML file.
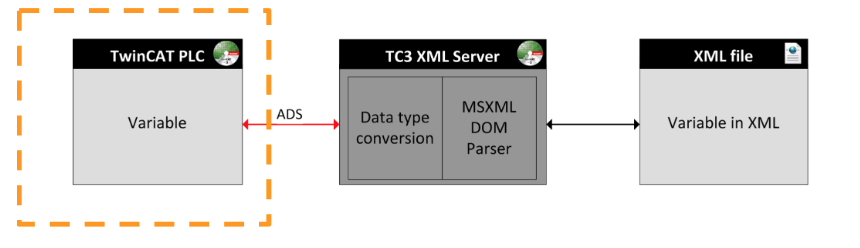
XML File
This is an XML File stored in TwinCAT Runtime.
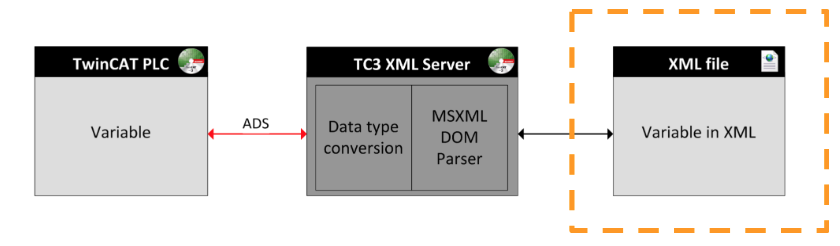
Principle of operation
The XML server communicates with the TwinCAT PLC via ADS All variables written by the XML server are converted to text and written to an XML file via the MSXML DOM parser The XML file does not contain any information about data types, only the variable names and values as tag names only as tag names.
<variable name> value </variable name> |
Conversely, during a read operation, the data type of the variable being read is transferred to the XML Server via ADS and the text from the XML file is converted accordingly.
Data Type
This is an example of the correspondence between TwinCAT PLC Runtime variables and XML File.
Function Block
FB_XmlSrvRead
The Function Block FB_XmlSrvRead here can be used to initialize PLC variables with data from an XML file. In this case, the input variable sXPath must point to a valid node in the XML file specified by sFilePath.
VAR_INPUT
Variable | Data Type | Description |
sNetId | T_AmsNet_Id | Network address of the TwinCAT 3 XML server, empty string for Local PC. |
ePath | E_OpenPath | Specify the TwinCAT system path on the target device where the file will be opened |
nMode | WORD | You can control how XML files are evaluated, currently only XMLSRV_SKIPMISSING and XmlSrvRead are supported. |
pSymAddr | DWORD | Address Offset of the PLC variable to which the data from the XML file is written. |
cbSymSize | UDINT | Size of the PLC variable to which data from the XML file is written. |
sFilePath | T_MaxString | Specify the path and filename of the file that FB will open. Note that the path is only for the file system of the local computer, the path cannot be used on a network. |
sXPath | T_MaxString | This will be the address of the tag in the XML document from which the data is to be accessed. |
bExecute | BOOL | Execute FB with start-up signal. |
tTimeout | TIME | Maximum running time of FB |
VAR_OUTPUT
Variable | Data Type | Description |
bBusy | BOOL | True=FB in progress |
bError | BOOL | True= FB error exists |
nErrId | UDINT | Error code returned by TC3 XML Server |
FB_XmlSrvReadByName
You can use FB_XmlSrvReadByName here to initialize PLC variables with data from an XML file. In this case, the input variable sXPath must point to a valid node in the XML file specified by sFilePath.
VAR_INPUT
Variable | Data Type | Description |
sNetId | T_AmsNet_Id | The network address of the TwinCAT 3 XML server, which must be an empty string on the Local PC. |
ePath | E_OpenPath | Specify the TwinCAT system path on the target device to open the file |
nMode | WORD | You can control how XML files are evaluated, currently only XMLSRV_SKIPMISSING and XmlSrvRead are supported. |
sSymName | T_MaxString | This will be the name of the PLC symbol to which data from the XML file will be written. |
sFilePath | T_MaxString | Specify the path and file name of the file that FB will open. Note that the path is only for the local computer’s file system; the path cannot be used on a network. |
sXPath | T_MaxString | This will be the address of the tag in the XML document from which the data will be accessed. |
bExecute | BOOL | 立ち上げ信号でFBを実行する |
tTimeout | TIME | Maximum execution time of FB |
VAR_OUTPUT
Variable | Data Type | Description |
bBusy | BOOL | True=FB in progress |
bError | BOOL | True= FB error exists |
nErrId | UDINT | Error code returned by TC3 XML Server |
Installation
Download the XML Server Installation File for TF6421 from the Beckhoff website.
Start the TF6421 installer, select English >Ok to proceed.
Click Next> to continue.
Agree to the license and proceed with Next>.
Enter your personal information and proceed with Next>.
Select Complete and proceed with Next>.
Proceed with Next>.
Get started with Install.
Just a second..
Done!
Implementation
We will now use the TF6421 API from TwinCAT3 to read the data in the XML File.
Add Library
Add the Tc2_XMLDataSrv library to your project.
DUT
The next step is to create a structure that matches the structure of the XML File.
DUT_MyXML
TYPE DUT_MyXML : STRUCT COMMON:STRING(30); BOTANICAL:STRING(30); ZONE:INT; LIGHT:STRING(30); PRICE:REAL; AVAILABILITY:BOOL; END_STRUCT END_TYPE |
DUT_MyXML_Books
TYPE DUT_MyXML_Books : STRUCT title:STRING(30); author:STRING(30); year:DINT; price:REAL; END_STRUCT END_TYPE |
DUT_MyXML and DUT_MyXML_Books do not need to worry about variable names, as long as they actually match the XML File, data type, and order.
Program
VAR
This one defines variables to be used in the program.
PROGRAM MAIN VAR fbXMLSrvRead:FB_XmlSrvRead; fbXmlSrvReadByName:FB_XmlSrvReadByName; bExecute:BOOL; bReset :BOOL; sFilePath:T_MaxString:=’C:\Users\threespc02\OneDrive\Desktop\plant_catalog-1.xml’; sXMLPath:T_MaxString:=’/CATALOG/PLANT’; sXMLPath2:T_MaxString:=’/CATALOG/book’; iState:INT:=0; myXMLValue:DUT_MyXML; myXMLValueReadByName:DUT_MyXML_Books; ErrorCode:UDINT; END_VAR |
This is the XML File used in this article, and the setting corresponds to a variable such as sFilePath.
Code
This program reads an XML File and reads the data in it using fbXmlSrvReadByName and fbXMLSrvRead Function Block.
CASE iState OF 0: fbXMLSrvRead( pSymAddr:=ADR(myXMLValue) ,cbSymSize:=SIZEOF(myXMLValue) ,sFilePath:=sFilePath ,sXPath:=sXMLPath ,bExecute:=FALSE ); fbXmlSrvReadByName( sSymName:=’MAIN.myXMLValueReadByName’ ,sFilePath:=sFilePath ,sXPath:=sXMLPath2 ,bExecute:=FALSE ); ErrorCode:=0; IF NOT fbXMLSrvRead.bBusy AND NOT fbXMLSrvRead.bError AND NOT fbXmlSrvReadByName.bBusy AND NOT fbXmlSrvReadByName.bError AND bExecute THEN iState:=10; END_IF 10: fbXMLSrvRead( bExecute:=TRUE ); IF fbXMLSrvRead.bBusy AND NOT fbXMLSrvRead.bError THEN iState:=20; END_IF; IF fbXMLSrvRead.bError THEN iState:=900; END_IF 20: fbXMLSrvRead( bExecute:=FALSE ); IF NOT fbXMLSrvRead.bBusy THEN iState:=100; END_IF ; 100: fbXmlSrvReadByName( bExecute:=TRUE ); IF fbXmlSrvReadByName.bBusy AND NOT fbXmlSrvReadByName.bError THEN iState:=110; END_IF; IF fbXmlSrvReadByName.bError THEN iState:=910; END_IF 110: fbXmlSrvReadByName(bExecute:=FALSE); IF NOT fbXmlSrvReadByName.bBusy THEN iState:=120; END_IF 120: bExecute:=FALSE; iState:=0; 900: ErrorCode:=fbXMLSrvRead.nErrId; bExecute:=FALSE; IF bReset THEN iState:=0; END_IF 910: ErrorCode:=fbXmlSrvReadByName.nErrId; bExecute:=FALSE; IF bReset THEN iState:=0; END_IF ; END_CASE |
Error 1828
If error 1828 occurs, you will need to enter your license.
Open SYSTEM>License.
This is the TwinCAT3 license management screen.
Check in TF6421.
The license for TF6421 is now in Missing status, please download Hardware Configuration one more time.
Result
Done!XML File data has been loaded.
Download
You can download the article project from this link.
https://github.com/soup01Threes/TwinCAT3/blob/main/Project_TF6421_XML_ReadExample.rar