The Beckhoff TwinCAT PLC library Tc3_JsonXml can be used to create JSON and XML documents. In this example we will use Tc3_JsonXml to create a JSON Object.
Let’s start!
SAX (simple API for XML)
SAX was originally developed to handle XML documents, but can also be used for other data formats such as JSON.
DOM (Document Object Model)
The DOM is a specification for accessing XML documents, but it can also be used for other data formats such as HTML and JSON.
JSON document
Here is an example of a JSON document.
{ “VariableNameX”: 0.0, “VariableNameY”: 0.0, “VariableNameZ”: 0.0 } |
Metadata
The Tc3_JsonXml library contains a function block FB_JsonReadWriteDataType that allows automatic generation of metadata by PLC attributes.
{ “Values”: { “VariableNameX”: 0.0, “VariableNameY”: 0.0, “VariableNameZ”: 0.0 }, “MetaData”: { “VariableNameX”: { “Unit”: “A” }, “VariableNameY”: { “Unit”: “V” }, “VariableNameZ”: { “Unit”: “mA” } } } |
Function Block
FB_JsonSaxWriter
The STRING type variables used here in this Function Block are based on the UTF-8 format ,and are as common in MQTT communications as in JSON documents.
AddKey
This method adds a new property key to the current position of the SAX writer. The value of the new property is usually set subsequently.
For example, you can create the value by using the following Method.
- AddBase64
- AddBool
- AddDateTime
- AddDcTime
- AddDint
- AddFileTime
- AddHexBinary
- AddLint
- AddLreal
- AddNull
- AddRawArray
- AddRawObject
- AddReal
- AddString
- AddUdint
- AddUlint.
Syntax
The Input parameter is a string that adds a new property key at the current position.
METHOD AddKey VAR_IN_OUT CONSTANT key : STRING; END_VAR |
Example
The following example adds a new property key “‘PropertyName'” at the current position.
fbJson.AddKey(‘PropertyName’); |
AddReal
This method adds a value of data type REAL to a property. Note that it must be pre-created with the method AddKey().
Syntax
The Input parameter is a REAL variable that adds a new value to the current position.
METHOD AddReal VAR_INPUT value : REAL; END_VAR |
Example
The following example adds a new property key “‘PropertyName'” and 42.42 value at the current position.
fbJson.AddKey(‘PropertyName’); fbJson.AddReal(42.42); |
AddDint
This method adds a value of DINT data type to a property. Typically, the corresponding property is pre-created with the method AddKey().
Syntax
The Input parameter is a DINT variable that adds a new value to the current position.
METHOD AddDint VAR_INPUT value : DINT; END_VAR |
Example
The following example adds a new property key “‘nNumber'” and 42 values to the current position.
fbJson.AddKey(‘nNumber’); fbJson.AddDint(42); |
StartArray
This method generates a new JSON array start position array symbol [ ] and inserts it at the current position of the SAX writer.
Syntax
The Input parameter is a REAL variable that adds a new array at the current position.
METHOD StartArray : HRESULT |
Example
The following example adds a new array at the current position.
fbJson.StartArray(); |
AddRawArray
This method adds a JSON array to the specified property as a value. It also requires that a SAX writer be in the corresponding valid position.
- Immediately after the AddKey()
- Immediately after the StartArray()
- After the ResetDocument()
Syntax
METHOD AddRawArray VAR_IN_OUT CONSTANT rawJson : STRING; END_VAR |
Example
The following example adds a new Json array “‘PropertyName'” at the current position, with Elements 1,2,4.
fbJson.AddKey(‘PropertyName’); fbJson.AddRawArray(‘1, 2, 4’); |
EndArray
This method generates a “]” at the end of the started JSON array and inserts it at the current position of the SAX writer.
Syntax
METHOD EndArray : HRESULT Sample call: |
Example
Below is an example of EndArra() usage.
fbJson.EndArray(); |
StartObject
This method creates a new JSON object ” { ” and inserts it into the current position of the SAX writer.
Syntax
METHOD StartObject : HRESULT |
Sample call
The following example shows the use of StartObject().
fbJson.StartObject(); |
EndObject
This method generates the initiated JSON object ” }” and inserts it at the current position of the SAX writer.
Syntax
METHOD EndObject : HRESULT |
Sample call
The following example illustrates the use of EndObject().
fbJson.EndObject(); |
ResetDocument
This method resets the JSON object currently created by the SAX writer.
Syntax
METHOD ResetDocument : HRESULT |
Sample call
Below is an example ResetDocument() usage.
fbJson.ResetDocument(); |
GetDocument
This method returns the JSON object currently being created in SAX Writer with data type STRING(255).
Note that the maximum size of the string returned by GetDocument() is 255 characters. For strings larger than that, the method returns a NULL string and the CopyDocument() method must be used.
Syntax
METHOD GetDocument : STRING(255) VAR_OUTPUT hrErrorCode: HRESULT; END_VAR |
Sample call
Below is an example of GetDocument() usage.
sTargetString := fbJson.GetDocument(); |
Example1
Example 1 creates a JSON Object with only one real number.
Program
VAR fbJson : FB_JsonSaxWriter; jsonDoc ,jsonDoc1 :SJsonValue; bnew:BOOL; Examples:ARRAY[0..99]OF BOOL; sJsonDoc:STRING(2000); r32:REAL; END_VAR IF Examples[1] THEN r32:=r32+0.0002; IF r32 > 10000 THEN r32:=0.0; END_IF fbJson.StartObject(); fbJson.AddKey(‘Sensor1’); fbJson.AddReal(r32); fbJson.EndObject(); sJsonDoc := fbJson.GetDocument(); fbJson.ResetDocument(); END_IF |
Result
If the Method continues to run, the JSON Object is constantly being updated.
The string sJSONDoc can be converted to a JSON OBJECT and is still being updated.
In fact, StartObject and others use this Method to generate JSON Objects like this.
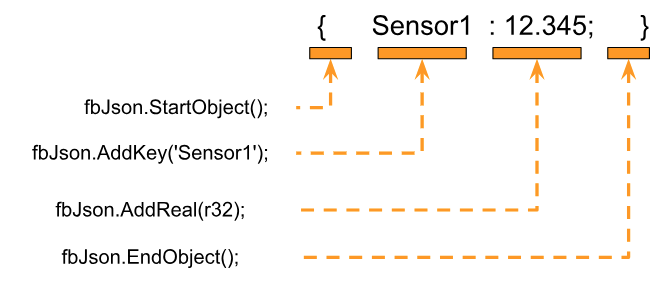
Example2 Array
Example2 creates a JSON Object with one real number, a real number array, and an integer array.
Program
IF Examples[2] THEN r32:=r32+0.0002; IF r32 > 10000 THEN r32:=0.0; END_IF fbJson.StartObject(); fbJson.AddKey(‘Sensor1’); r32:=r32+0.0002; fbJson.AddReal(r32); fbJson.AddKey(‘r32Array’); fbJson.StartArray(); fbJson.AddRawArray(‘123.1,444.5,512.3’); fbJson.EndArray(); fbJson.AddKey(‘iArray’); fbJson.StartArray(); fbJson.AddRawArray(‘123,1,5,6,7,8,9,0,111’); fbJson.EndArray(); fbJson.EndObject(); sJsonDoc := fbJson.GetDocument(); fbJson.ResetDocument(); END_IF |
Result
The string sJSONDoc can be converted to a JSON OBJECT and is still being updated.
In fact, StartObject and others use this Method to generate JSON Objects like this.
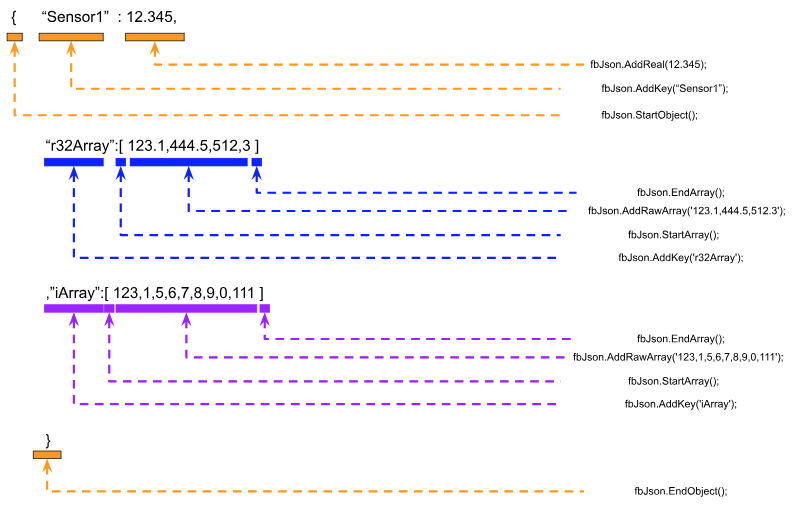
Exmaple3 Add Object
Example3 creates a JSON Object that contains not only one real number, one real array, and one integer array, but also one Object.
Program
IF Examples[3] THEN r32:=r32+0.0002; IF r32 > 10000 THEN r32:=0.0; END_IF fbJson.StartObject(); fbJson.AddKey(‘Sensor1’); fbJson.AddReal(r32); fbJson.AddKey(‘r32Array’); fbJson.StartArray(); fbJson.AddRawArray(‘123.1,444.5,512.3’); fbJson.EndArray(); fbJson.AddKey(‘iArray’); fbJson.StartArray(); fbJson.AddRawArray(‘123,1,5,6,7,8,9,0,111’); fbJson.EndArray(); fbJson.AddKey(‘MotorData’); fbJson.StartObject(); fbJson.AddKey(‘Speed’); fbJson.AddReal(123.4); fbJson.AddKey(‘Position’); fbJson.AddReal(512.1); fbJson.AddKey(‘ErrorCode’); fbJson.AddDint(1234); fbJson.EndObject(); fbJson.EndObject(); sJsonDoc := fbJson.GetDocument(); fbJson.ResetDocument(); END_IF; |
Result
The string sJSONDoc can be converted to a JSON OBJECT and is still being updated.
In fact, StartObject and others use this Method to generate JSON Objects like this.
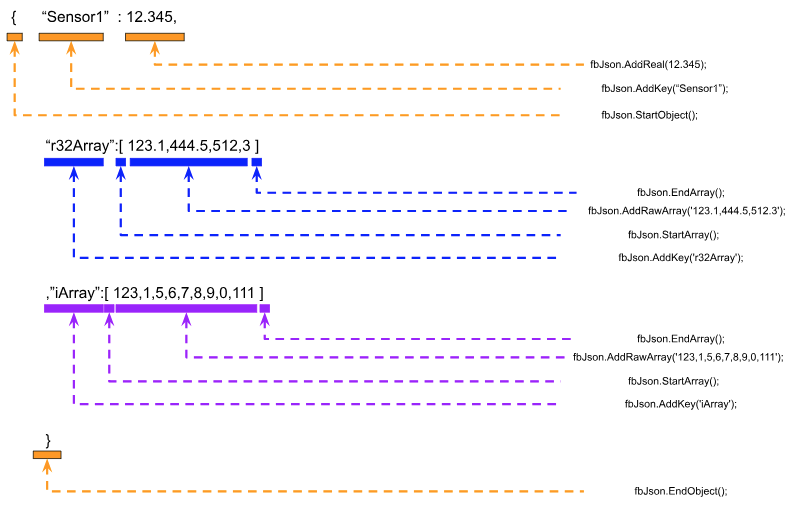