This article I use the Beckhoff TwinCAT’s Automation interface to create a project from the TwinCAT IDE Automatically . Beckhoff’s InfoSys has some explanation, but this article is a bit of a compliment about this. Let’s Start!
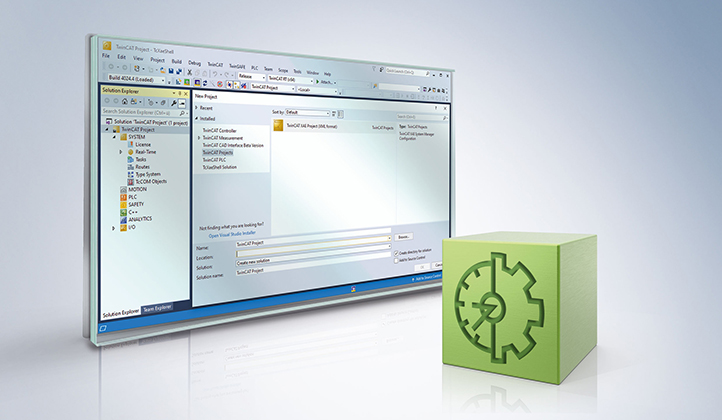
TwinCAT Automation Interface?
TwinCAT Automation Interface allows TwinCAT User to automatically create programs and operate tools via API. It can be implemented from a language (Windows PowerShell/IronPython/VBScript, etc.). In this article, I have implemented PLC project creation, Profinet Controller and Devices addition, and Import the GVL.
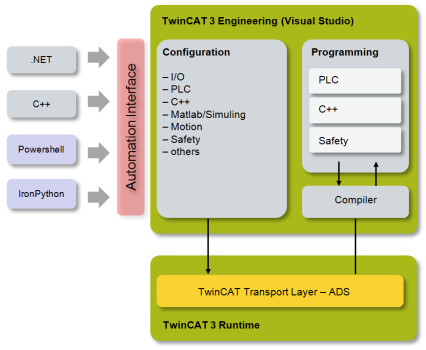
This article I use the Beckhoff TwinCAT’s Automation interface to create a project from the TwinCAT IDE Automatically . Beckhoff’s InfoSys has some explanation, but this article is a bit of a compliment about this. Let’s Start!
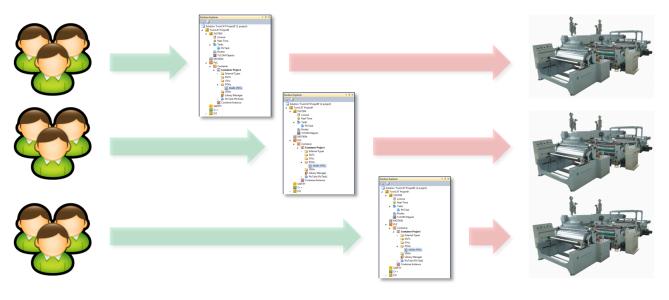
TwinCAT Automation Interface?
TwinCAT Automation Interface allows TwinCAT User to automatically create programs and operate tools via API. It can be implemented from a language (Windows PowerShell/IronPython/VBScript, etc.). In this article, I have implemented PLC project creation, Profinet Controller and Devices addition, and Import the GVL.
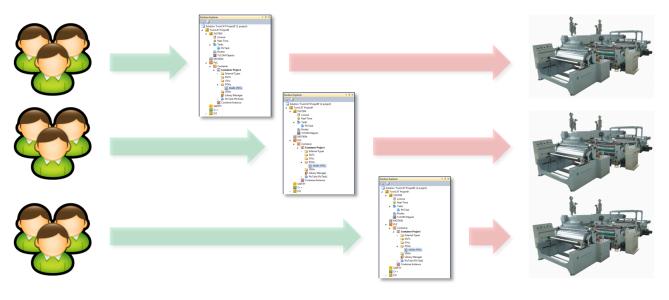
In the conventional method, projects are generally created manually. This approach is error prone and time consuming.
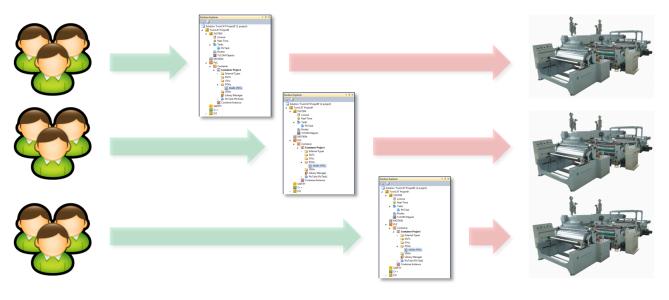
By using the TwinCAT Automation Interface, the Hardware-Configuration of the TwinCAT project and the import of programs are automatically created and can be adapted for different applications.
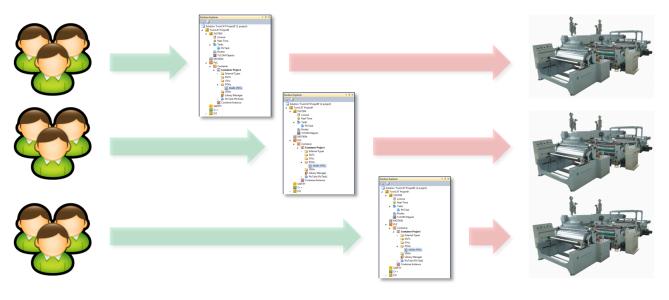
System Requirment
Note the Automaton Interface engineer PC and the actual IPC TwinCAT Runtime version must be the same or higher than the engineer PC version.
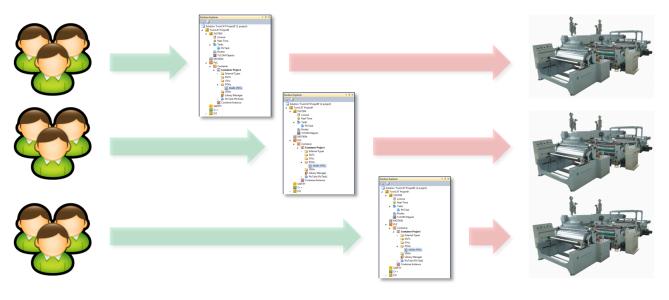
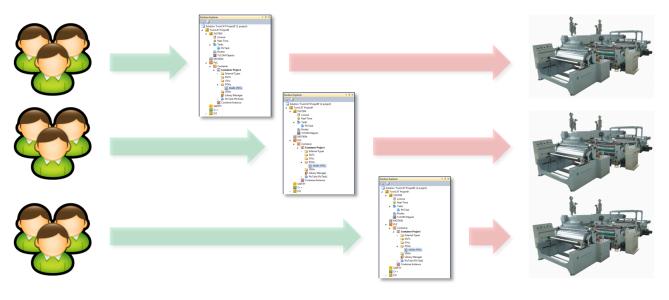
And the Script of Automation interface can be executed on 32Bit/64Bit Platforms, but please be sure to compile and execute the compiler as 32 Bit Mode.
TwinCAT Version
TwinCAT Version 3.1 Build 4020.0 or higher is required.
Programming Language
C# and Visual Basic .NET is recommended.
Create Console Apps
Start your Visual Studio.
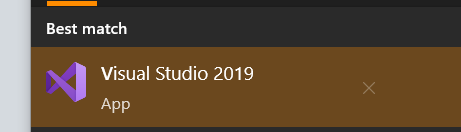
Create a new project.
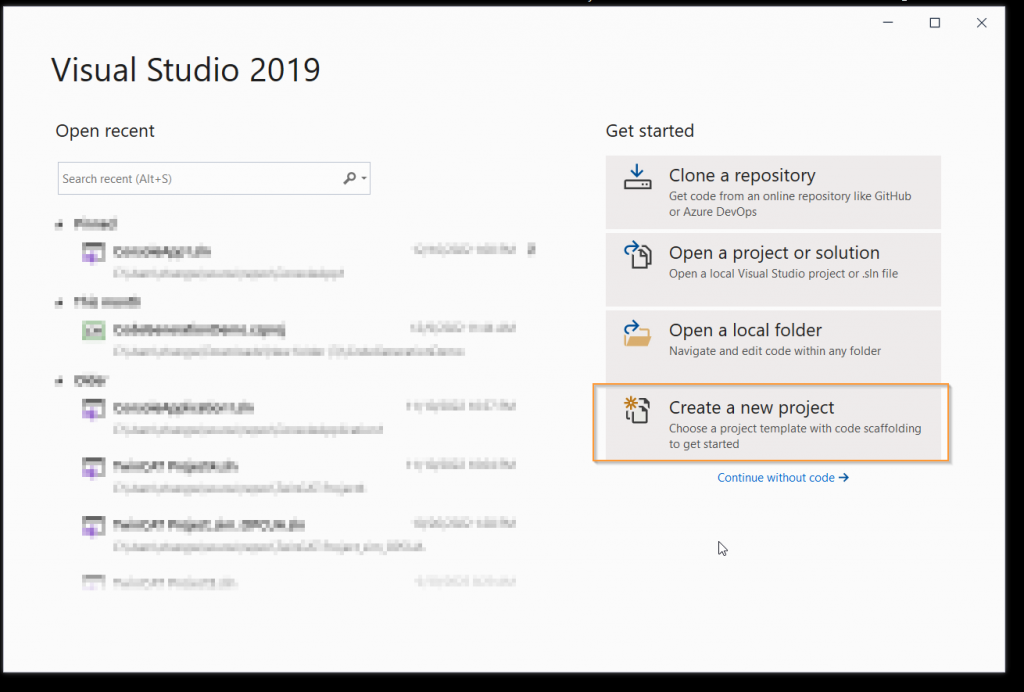
Insert a Console Application C# application.
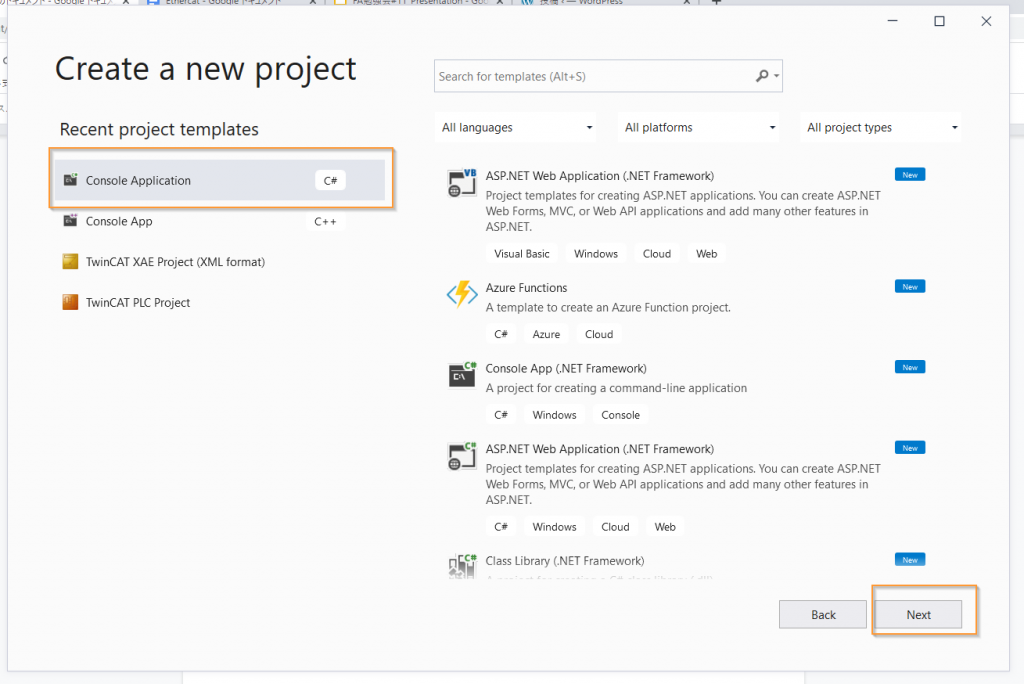
Configure your project name and Next.
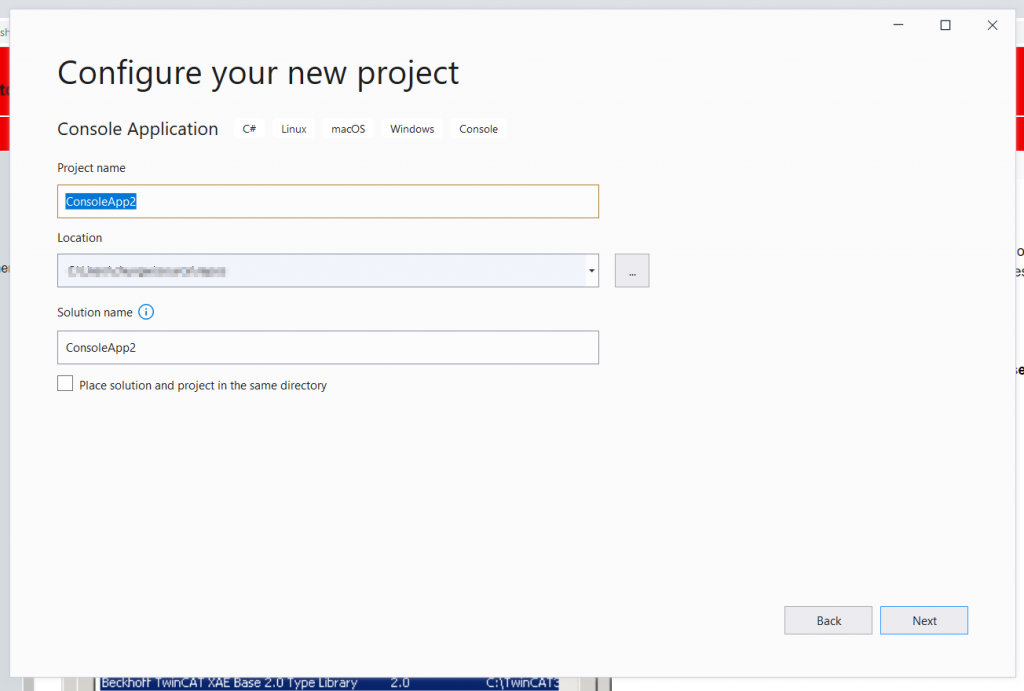
Installation
Before using the automation interface, a little groundwork is still required.
.NET packages
Please download and install the .NET Packages.
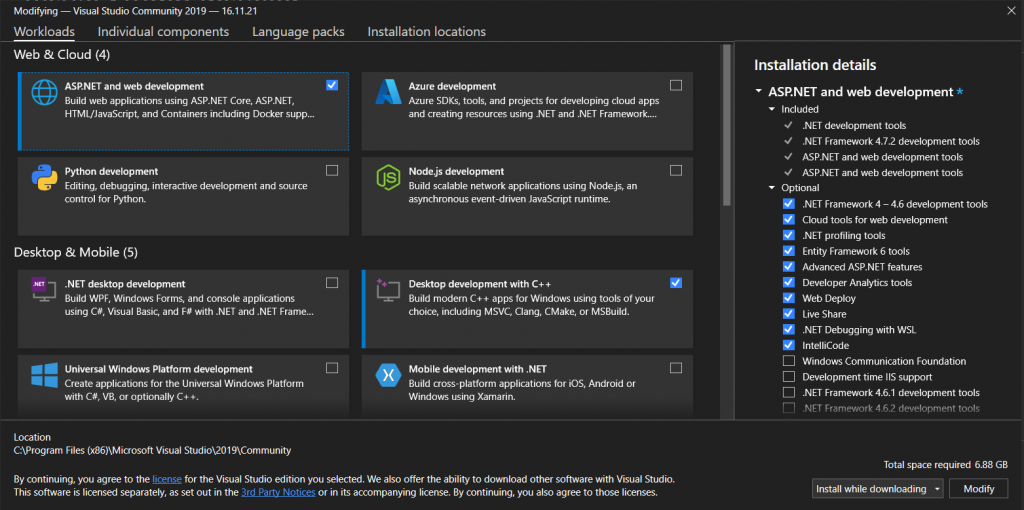
nugetpackages
If you are using VS with version lower than 207, please download and install the nuget packages.
https://www.nuget.org/downloads
envdte packages
Go to Browse and search EnvDTE to install this package.
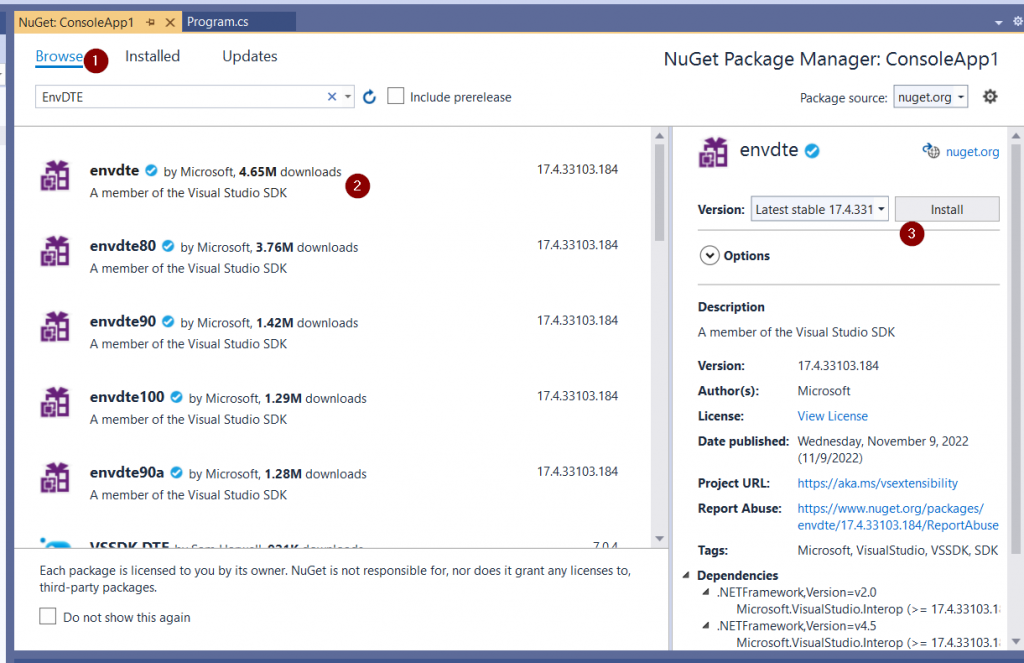
Ok.
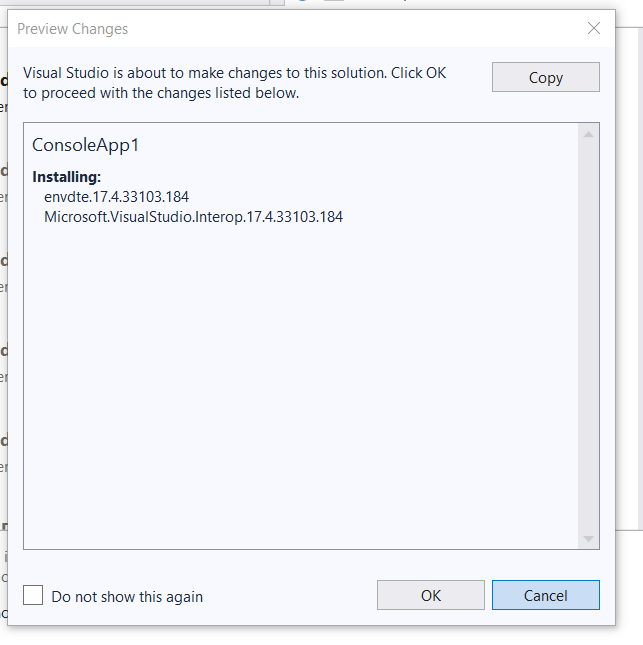
Agree the License.
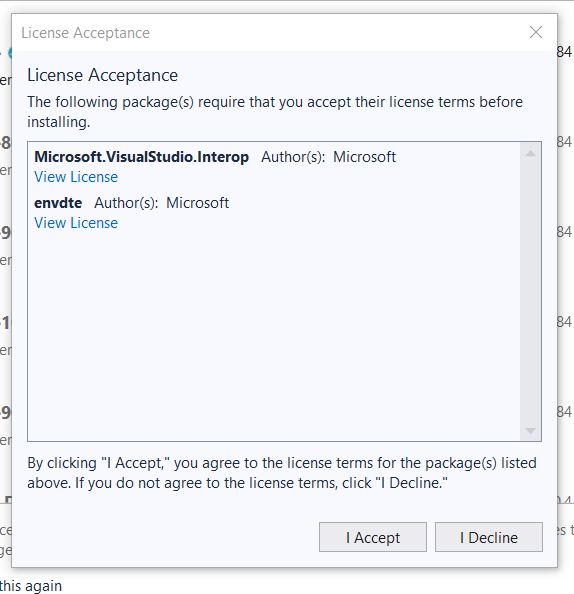
Done!
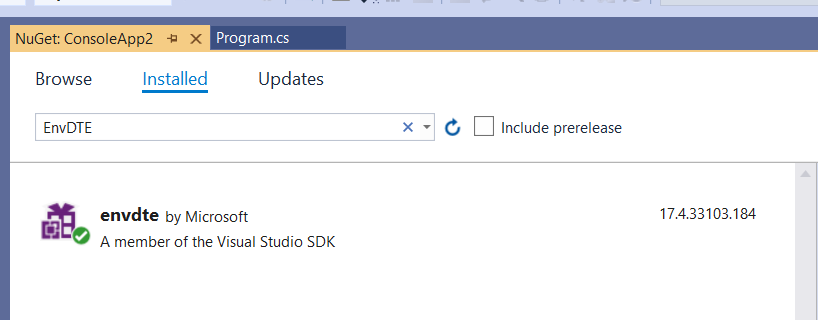
Add Reference
Now we need to add the TwinCAT Type Library Reference into our C# Project.
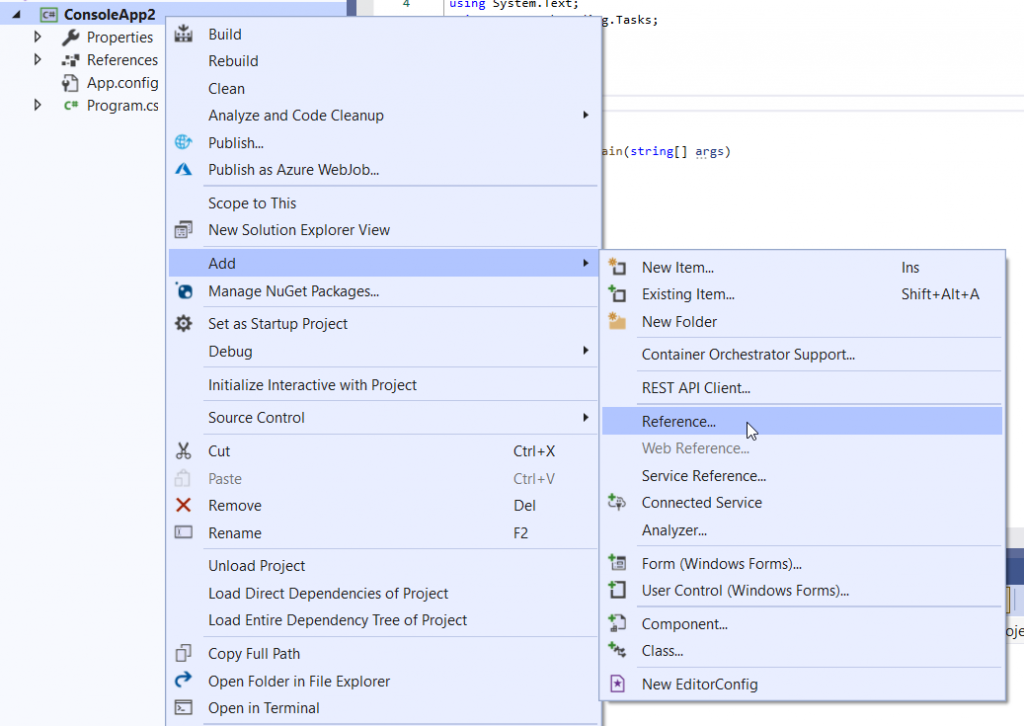
Check the Beckhoff TwinCAT XAE Base 3.3 Type Library and press OK.
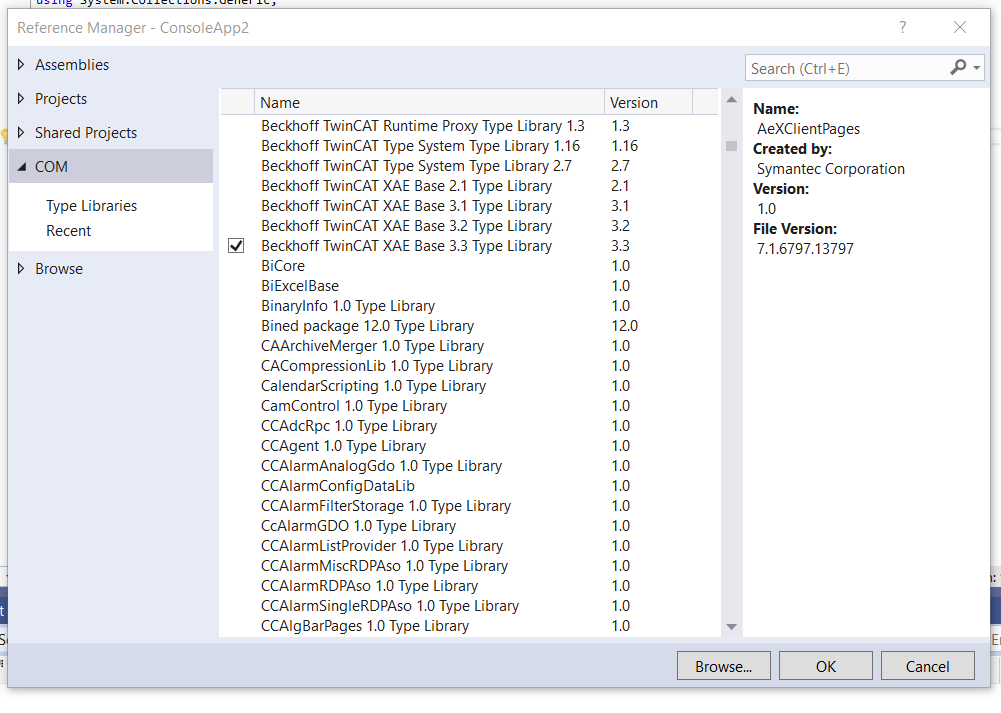
Check your dte Version
Before you start to program with Automation Interface, you need to check the version of Visual Studio DTE object.Go to egistry Editor>HKEY_CLASSES_ROOT and VisualStudo.DTE.16.0 is used in my OS.
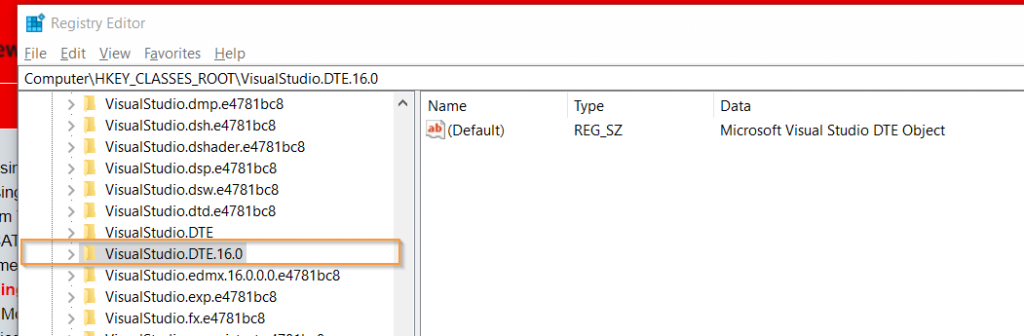
Implementation-1 Create the TwinCAT Project
In the Implementation1 I will show you how to use TwinCAT Automation Interface to Create a TwinCAT Project with C#.
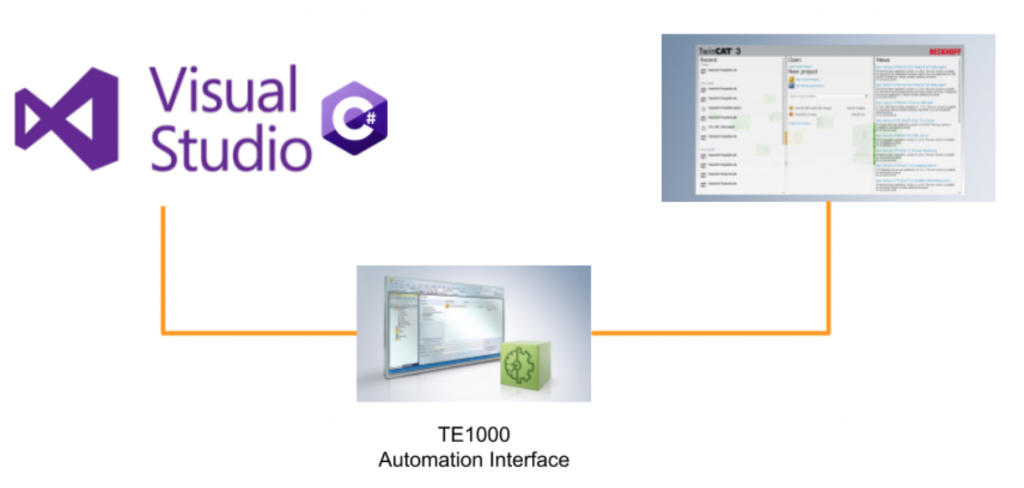
program
using System; using System.IO; using TCatSysManagerLib; namespace ConsoleApp2 { class Program { static string getTheCurrentDataTime() { DateTime dt = DateTime.Now; return dt.ToString(“yyyy/MM/dd HH:mm:ss”); } static void Main(string[] args) { //Init String MyStandardPLCProjectTemplates = “Standard PLC Template.plcproj”; String PLCName = “MyPLC”; String path = @”C:\Users\chungw\Downloads\Myp”; int S210Telegram30 = 5; int S210Telegram3 = 7; //Creating the Visutal Studio DTE Console.WriteLine(“{0}:Getting VisualStudio DTE ID…”, getTheCurrentDataTime()); Type t = System.Type.GetTypeFromProgID(“VisualStudio.DTE.16.0”); Console.WriteLine(“{0}:VisualStudio DTE ID is read!”, getTheCurrentDataTime()); //Create the Instance Console.WriteLine(“{0}:Creating the Instance..”, getTheCurrentDataTime()); EnvDTE.DTE dte = (EnvDTE.DTE)System.Activator.CreateInstance(t); dte.SuppressUI = false; dte.MainWindow.Visible = true; Console.WriteLine(“{0}:Start to Create Folder..”, getTheCurrentDataTime()); DirectoryInfo di = new DirectoryInfo(path); di.Create(); dte.Solution.Create(path, “MySolution1”); dte.Solution.SaveAs(@”C:\Users\chungw\Downloads\Myp\Solution1.sln”); //Add Solution string template = @”C:\TwinCAT\3.1\Components\Base\PrjTemplate\TwinCAT Project.tsproj”; ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; } } } |
Result
Visual Studio is launched by Automation Interface and a TwinCAT project is created.
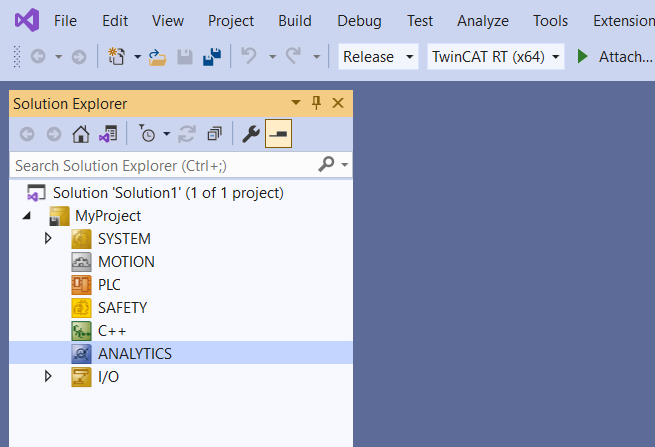
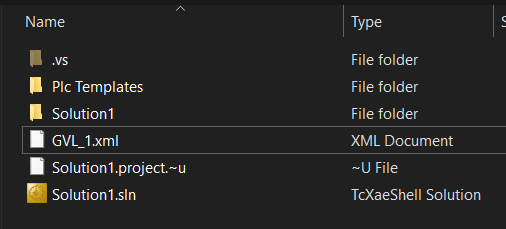
ITcSysManager
ITcSysManager is the Main interface of TwinCAT Automation Interface and it provides all the basic operation of TwinCAT.
Here is the code that uses the DTE Interface to create a new Visual Studio Project.
ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; |
Implementation-2 Add PLC Project
In this Implementation2, I will show you how to Create a Standard PLC Project but just not launch the Visual Studio.
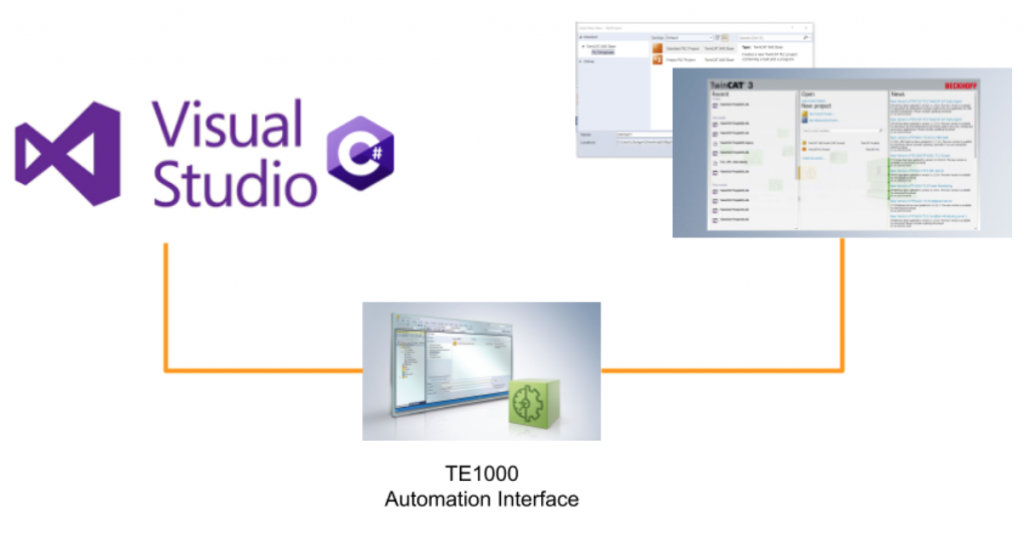
program
using System; using System.IO; using TCatSysManagerLib; namespace ConsoleApp2 { class Program { static string getTheCurrentDataTime() { DateTime dt = DateTime.Now; return dt.ToString(“yyyy/MM/dd HH:mm:ss”); } static void Main(string[] args) { //Init String MyStandardPLCProjectTemplates = “Standard PLC Template.plcproj”; String PLCName = “MyPLC”; String path = @”C:\Users\chungw\Downloads\Myp”; int S210Telegram30 = 5; int S210Telegram3 = 7; //Creating the Visutal Studio DTE Console.WriteLine(“{0}:Getting VisualStudio DTE ID…”, getTheCurrentDataTime()); Type t = System.Type.GetTypeFromProgID(“VisualStudio.DTE.16.0”); Console.WriteLine(“{0}:VisualStudio DTE ID is read!”, getTheCurrentDataTime()); //Create the Instance Console.WriteLine(“{0}:Creating the Instance..”, getTheCurrentDataTime()); EnvDTE.DTE dte = (EnvDTE.DTE)System.Activator.CreateInstance(t); dte.SuppressUI = false; dte.MainWindow.Visible = true; Console.WriteLine(“{0}:Start to Create Folder..”, getTheCurrentDataTime()); DirectoryInfo di = new DirectoryInfo(path); di.Create(); dte.Solution.Create(path, “MySolution1”); dte.Solution.SaveAs(@”C:\Users\chungw\Downloads\Myp\Solution1.sln”); //Add Solution string template = @”C:\TwinCAT\3.1\Components\Base\PrjTemplate\TwinCAT Project.tsproj”; ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; //PLC Console.WriteLine(“{0}:TwinCAT is started.”, getTheCurrentDataTime()); ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”); Console.WriteLine(“{0}:Creating PLC…”, getTheCurrentDataTime()); ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); //Get PLC Project ITcSmTreeItem plcProject = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project”); ITcPlcIECProject importExport = (ITcPlcIECProject)plcProject; importExport.PlcOpenImport(@”C:\Users\chungw\Downloads\Myp\GVL_1.xml”, (int)PLCIMPORTOPTIONS.PLCIMPORTOPTIONS_NONE); } } } |
Result
As you see, not only the Visual Studio is started up but a Standard PLC Project is inserted in our TwinCAT project.
.
And inside the Standard PLC Project, a Global Variables List that is named GVL_1 is created.
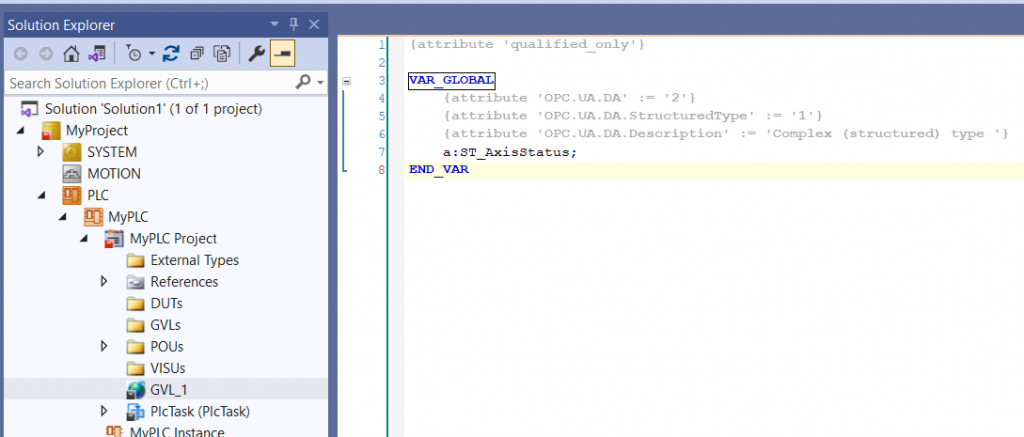
This Code is using the DTE Interface to create a TwinCAT Standard PLC Project, and that is a Template parameter inside.
//PLCConsole.WriteLine(“{0}:TwinCAT is started.”, getTheCurrentDataTime());ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”);Console.WriteLine(“{0}:Creating PLC…”, getTheCurrentDataTime());ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); |
There is also a folder named “Plc Templates” in my Solution directory also.
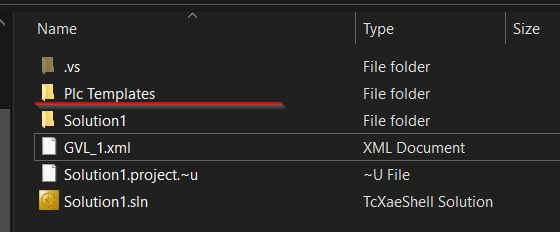
Inside that folder, you can find an empty PLC Template and Standard PLC Template are stored.
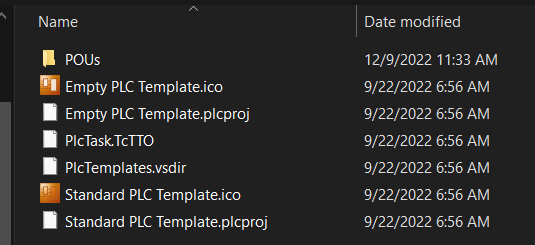
These templates are the same as the files that are stored in TwinCAT/3.1/Componentes/Plc/PlcTemplate/Plc Templates/.
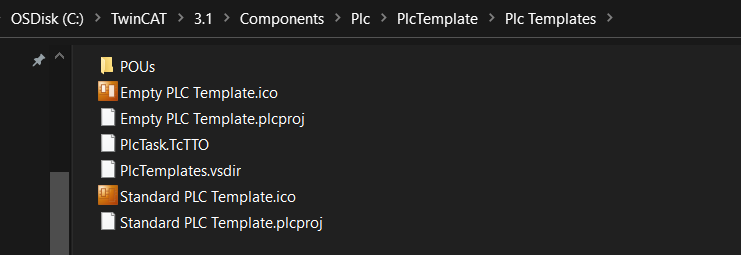
The operation is the same as while we create a PLC standard project in TwinCAT by using the GUI.
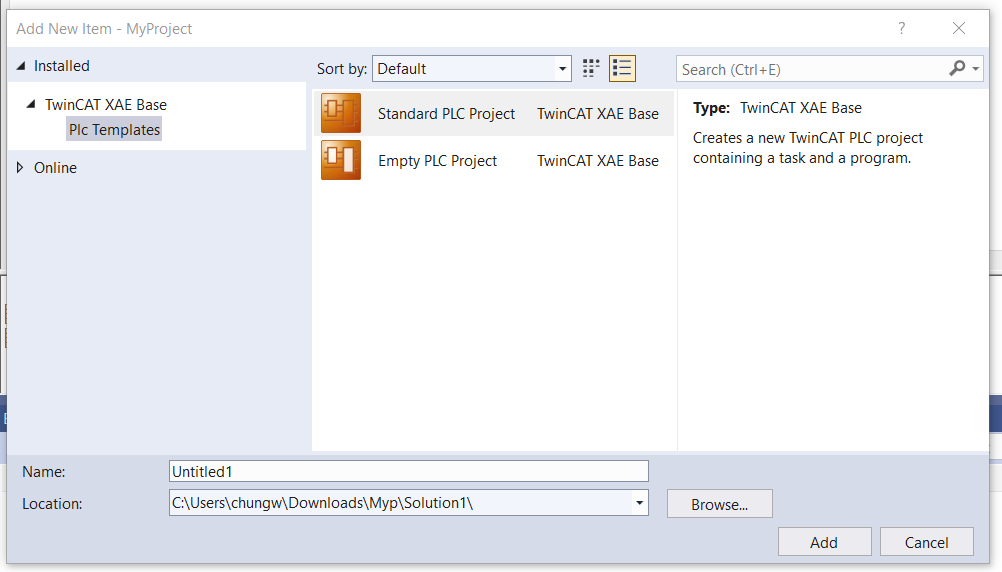
ITcSmTreeItem
In this Implementation ITcSmTreeItem is used and it can help you to get the Item inside your TwinCAT by using the LookIpTreeItem method.
ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”) |
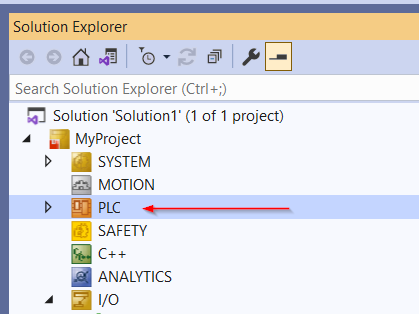
This line will trigger the Automation interface to create the PLC project with template setting.
ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); |
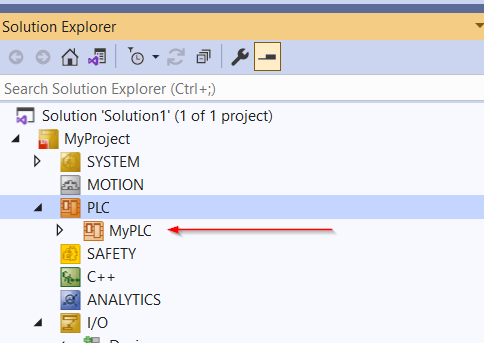
Finally , this line will get the PLC Project Object.
ITcSmTreeItem plcProject = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project”); |
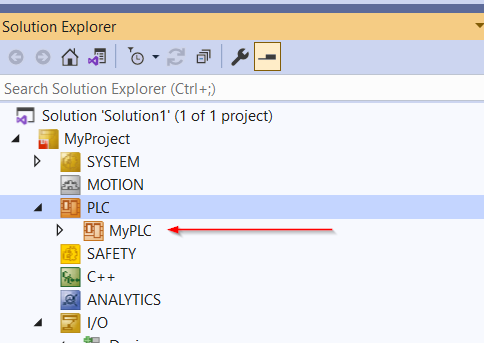
Do you know what this path is?
“TIPC^MyPLC^MyPLC Project” |
You can check the Properties of your Object in TwinCAT, and then there is a PathName item inside,separate with ^.It is the absolute path of your Object.
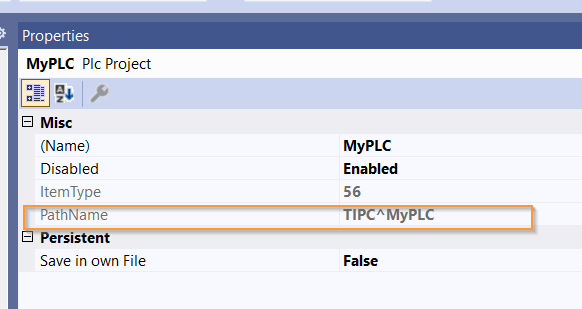
So, the path of this object is pointed to the MyPLC Project.
“TIPC^MyPLC^MyPLC Project” |
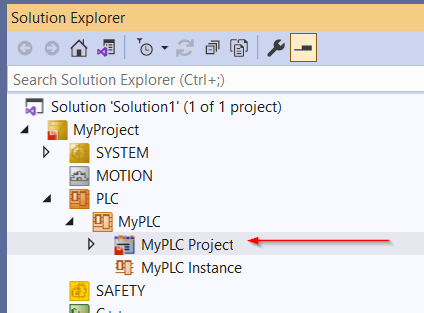
ITcPlcIECProject
In this Implementation I also use the automation interface to import the data from the PlcOpen XML Standard Format and make some library operations.
In TwinCAT,there are Import PLCopenXML・Export PLCopenXML・Save as Library・Save as library and install.
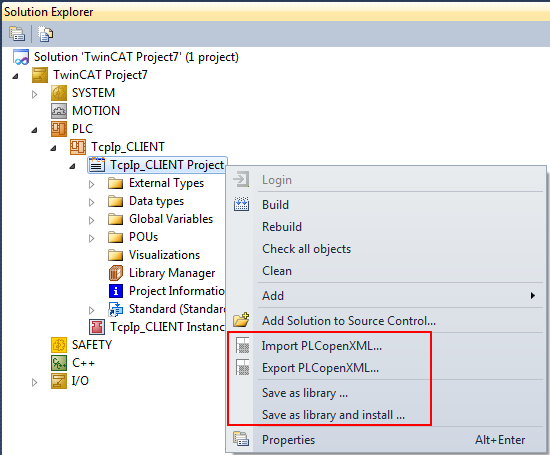
But In the TwinCAT Automation Interface you can only use PLCopenXML・Export PLCopenXML・Save as Library.
We import the GVL_1.xml file in the same directory to our PLC Project.
ITcPlcIECProject importExport = (ITcPlcIECProject)plcProject; importExport.PlcOpenImport(@”C:\Users\chungw\Downloads\Myp\GVL_1.xml”, (int)PLCIMPORTOPTIONS.PLCIMPORTOPTIONS_NONE); |
Implementation-3 import library
Now I will show you how to import the library by using Automation Interface.
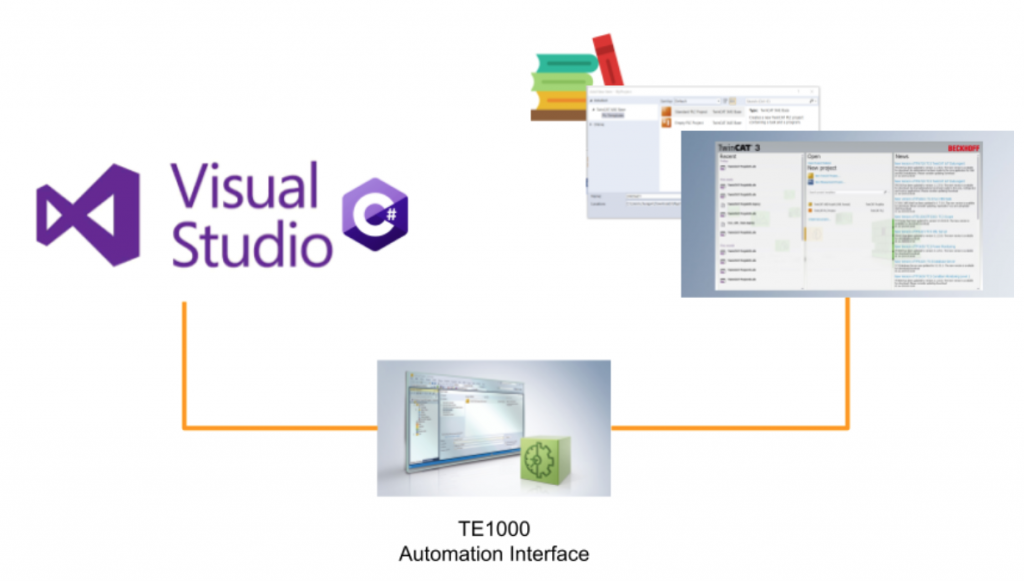
program
using System; using System.IO; using TCatSysManagerLib; namespace ConsoleApp2 { class Program { static string getTheCurrentDataTime() { DateTime dt = DateTime.Now; return dt.ToString(“yyyy/MM/dd HH:mm:ss”); } static void Main(string[] args) { //Init String MyStandardPLCProjectTemplates = “Standard PLC Template.plcproj”; String PLCName = “MyPLC”; String path = @”C:\Users\chungw\Downloads\Myp”; int S210Telegram30 = 5; int S210Telegram3 = 7; //Creating the Visutal Studio DTE Console.WriteLine(“{0}:Getting VisualStudio DTE ID…”, getTheCurrentDataTime()); Type t = System.Type.GetTypeFromProgID(“VisualStudio.DTE.16.0”); Console.WriteLine(“{0}:VisualStudio DTE ID is read!”, getTheCurrentDataTime()); //Create the Instance Console.WriteLine(“{0}:Creating the Instance..”, getTheCurrentDataTime()); EnvDTE.DTE dte = (EnvDTE.DTE)System.Activator.CreateInstance(t); dte.SuppressUI = false; dte.MainWindow.Visible = true; Console.WriteLine(“{0}:Start to Create Folder..”, getTheCurrentDataTime()); DirectoryInfo di = new DirectoryInfo(path); di.Create(); dte.Solution.Create(path, “MySolution1”); dte.Solution.SaveAs(@”C:\Users\chungw\Downloads\Myp\Solution1.sln”); //Add Solution string template = @”C:\TwinCAT\3.1\Components\Base\PrjTemplate\TwinCAT Project.tsproj”; ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; //PLC Console.WriteLine(“{0}:TwinCAT is started.”, getTheCurrentDataTime()); ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”); Console.WriteLine(“{0}:Creating PLC…”, getTheCurrentDataTime()); ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); //Get PLC Project ITcSmTreeItem plcProject = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project”); ITcPlcIECProject importExport = (ITcPlcIECProject)plcProject; //ITcSmTreeItem GVL = plcProject.CreateChild(“GVL_1”, 615, null, null); importExport.PlcOpenImport(@”C:\Users\chungw\Downloads\Myp\GVL_1.xml”, (int)PLCIMPORTOPTIONS.PLCIMPORTOPTIONS_NONE); //Reference Library ITcSmTreeItem references = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project^References”); ITcPlcLibraryManager libManager = (ITcPlcLibraryManager)references; libManager.AddLibrary(“Tc2_MC2”, “*”, “Beckhoff Automation GmbH”); libManager.AddLibrary(“Tc2_MC2_Drive”, “*”, “Beckhoff Automation GmbH”); } } } |
Result
As you see, Tc2_MC2 and Tc2_MC2_Drive libraries are imported into the TwinCAT Project.
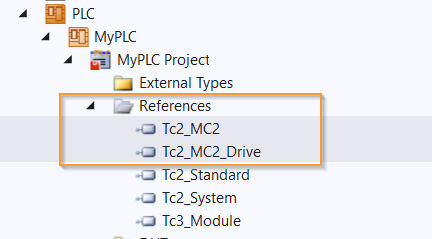
ITcPlcLibraryManager
ITcPlcLibraryManager is an object that allows you access the PLC library inside TwinCAT.
ITcPlcLibraryManager::AddLibrary is a method to add the library into your PLC project.
By using this method, parameters Name/Version/Company is necessary.
In this example, library Tc2_MC2 is used and the Name is Tc2_MC2,Version is * and Company is Beckhoff Automation GmbH.
ITcSmTreeItem references = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project^References”); libManager.AddLibrary(“Tc2_MC2”, “*”, “Beckhoff Automation GmbH”); libManager.AddLibrary(“Tc2_MC2_Drive”, “*”, “Beckhoff Automation GmbH”); |
Implementation-4 Create Profinet IO Controller
In this implementation,I will show you how to use Automation Interface to insert a Profinet IO Controller to the TwinCAT Project.
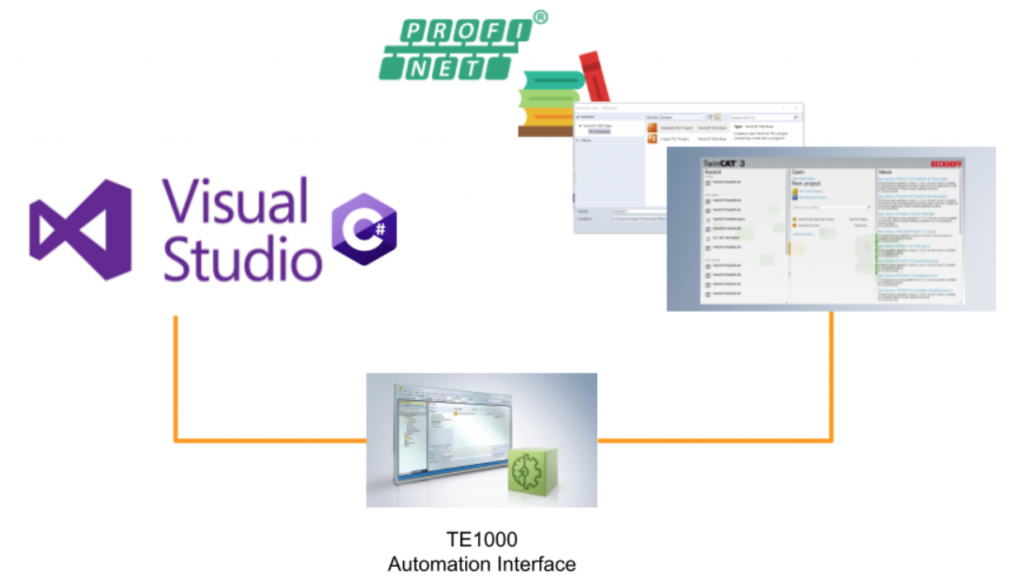
Program
using System; using System.IO; using TCatSysManagerLib; namespace ConsoleApp2 { class Program { static string getTheCurrentDataTime() { DateTime dt = DateTime.Now; return dt.ToString(“yyyy/MM/dd HH:mm:ss”); } static void Main(string[] args) { //Init String MyStandardPLCProjectTemplates = “Standard PLC Template.plcproj”; String PLCName = “MyPLC”; String path = @”C:\Users\chungw\Downloads\Myp”; int S210Telegram30 = 5; int S210Telegram3 = 7; //Creating the Visutal Studio DTE Console.WriteLine(“{0}:Getting VisualStudio DTE ID…”, getTheCurrentDataTime()); Type t = System.Type.GetTypeFromProgID(“VisualStudio.DTE.16.0”); Console.WriteLine(“{0}:VisualStudio DTE ID is read!”, getTheCurrentDataTime()); //Create the Instance Console.WriteLine(“{0}:Creating the Instance..”, getTheCurrentDataTime()); EnvDTE.DTE dte = (EnvDTE.DTE)System.Activator.CreateInstance(t); dte.SuppressUI = false; dte.MainWindow.Visible = true; Console.WriteLine(“{0}:Start to Create Folder..”, getTheCurrentDataTime()); DirectoryInfo di = new DirectoryInfo(path); di.Create(); dte.Solution.Create(path, “MySolution1”); dte.Solution.SaveAs(@”C:\Users\chungw\Downloads\Myp\Solution1.sln”); //Add Solution string template = @”C:\TwinCAT\3.1\Components\Base\PrjTemplate\TwinCAT Project.tsproj”; ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; //PLC Console.WriteLine(“{0}:TwinCAT is started.”, getTheCurrentDataTime()); ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”); Console.WriteLine(“{0}:Creating PLC…”, getTheCurrentDataTime()); ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); //Get PLC Project ITcSmTreeItem plcProject = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project”); ITcPlcIECProject importExport = (ITcPlcIECProject)plcProject; //ITcSmTreeItem GVL = plcProject.CreateChild(“GVL_1”, 615, null, null); importExport.PlcOpenImport(@”C:\Users\chungw\Downloads\Myp\GVL_1.xml”, (int)PLCIMPORTOPTIONS.PLCIMPORTOPTIONS_NONE); //Reference Library ITcSmTreeItem references = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project^References”); ITcPlcLibraryManager libManager = (ITcPlcLibraryManager)references; libManager.AddLibrary(“Tc2_MC2”, “*”, “Beckhoff Automation GmbH”); libManager.AddLibrary(“Tc2_MC2_Drive”, “*”, “Beckhoff Automation GmbH”); //Create the Profinet IO Controllers Console.WriteLine(“{0}:Createing the Profinet IO Controller..”, getTheCurrentDataTime()); ITcSmTreeItem io = sysManager.LookupTreeItem(“TIID”); ITcSmTreeItem ProfinetController = io.CreateChild(“PNIO Controller”, 113, null, null); ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><IP>#x0103a8c0</IP></IpSettings></DevicePnControllerDef></TreeItem>”); ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><Subnet>#x00ffffff</Subnet></IpSettings></DevicePnControllerDef></TreeItem>”); } } } |
Result
As you see, a PNIo Controller is inserted in your I/O Configuration.
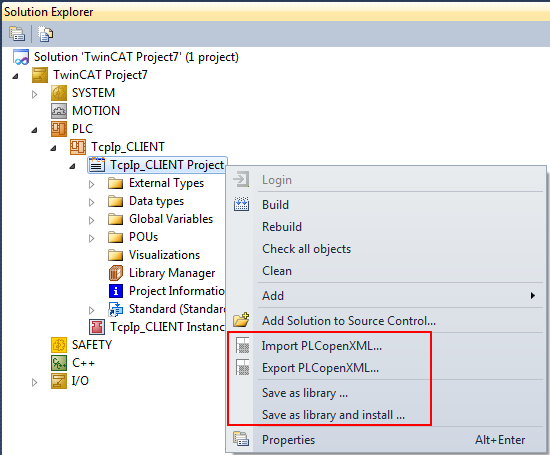
Almost all TwinCAT parts can be exported as XML, so you can select the part that needs to be exported>Extension>Selected Items>Export XML Description.
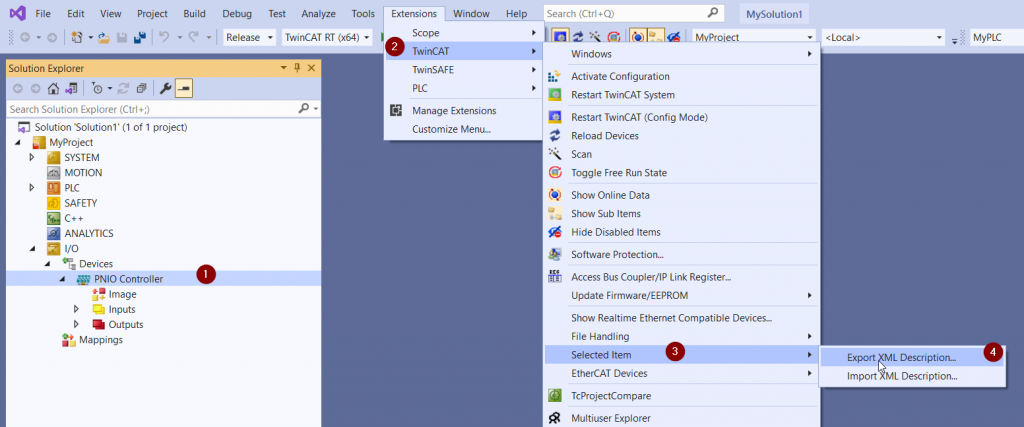
With LookupTreeItem(“TIID”) you can get the “I/O” Object of the TwinCAT Project. Once you get the I/O Object , not only Profinet Controller but also EtherCAT Master etc. can be added from CreateChild.
ITcSmTreeItem io = sysManager.LookupTreeItem(“TIID”); ITcSmTreeItem ProfinetController = io.CreateChild(“PNIO Controller”, 113, null, null); |
The number 113 corresponds to the Profinet Controller (RT).
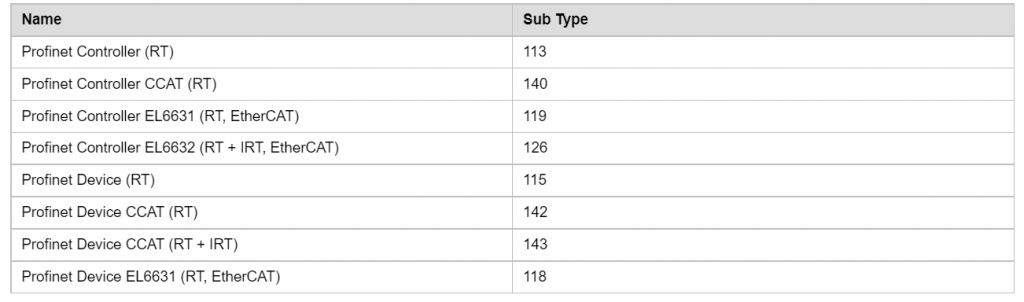
You can change the Object Property in the I/O Configuration from the ConsumeXml Method in the previous example. Do you remember the XML file that you exported? In fact, you can check the parameters that can be changed from the exported XML File.
For example,in this implementation we pass the parameter <TreeItem><DevicePnControllerDef><IpSettings><IP>#x0103a8c0</IP></IpSettings></DevicePnControllerDef></TreeItem>と<TreeItem><DevicePnControllerDef><IpSettings><Subnet>#x00ffffff</Subnet></IpSettings></DevicePnControllerDef></TreeItem>to ConsumeXml Method.
ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><IP>#x0103a8c0</IP></IpSettings></DevicePnControllerDef></TreeItem>”); ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><Subnet>#x00ffffff</Subnet></IpSettings></DevicePnControllerDef></TreeItem>”); |
Looking at the Export XML, for example, the name of the Profinet Controller in the TwinCAT Project is “PNIO Controller”.
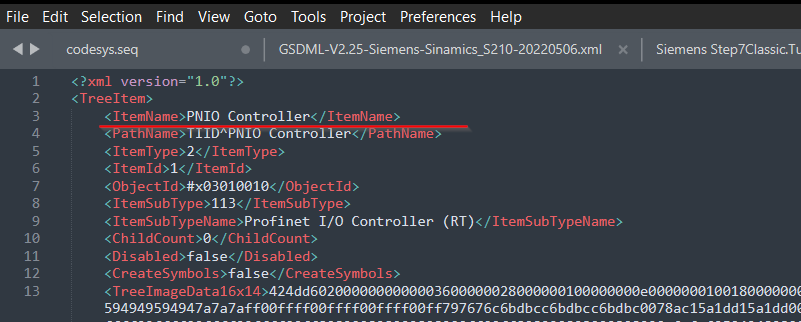
<IpSettings><IP>#x0103a8c0</IP></IpSettings> is actually an operation that changes the IP of the Controller. #x0103a8c0 can be encoded to IP addresses like 01/03/168/192 when converted to decimal.
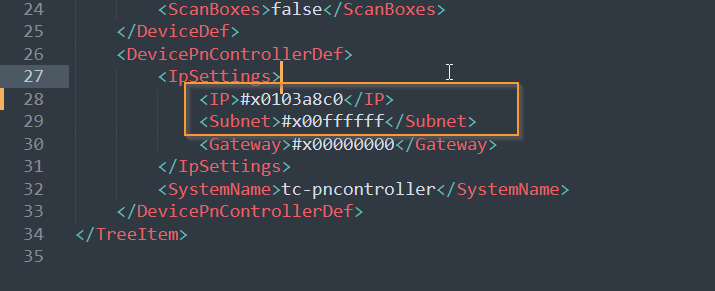
Implementation-5 Add S210
Finally, we will add Profinet Devices S210 under the Profinet Controller in Implementation-5.
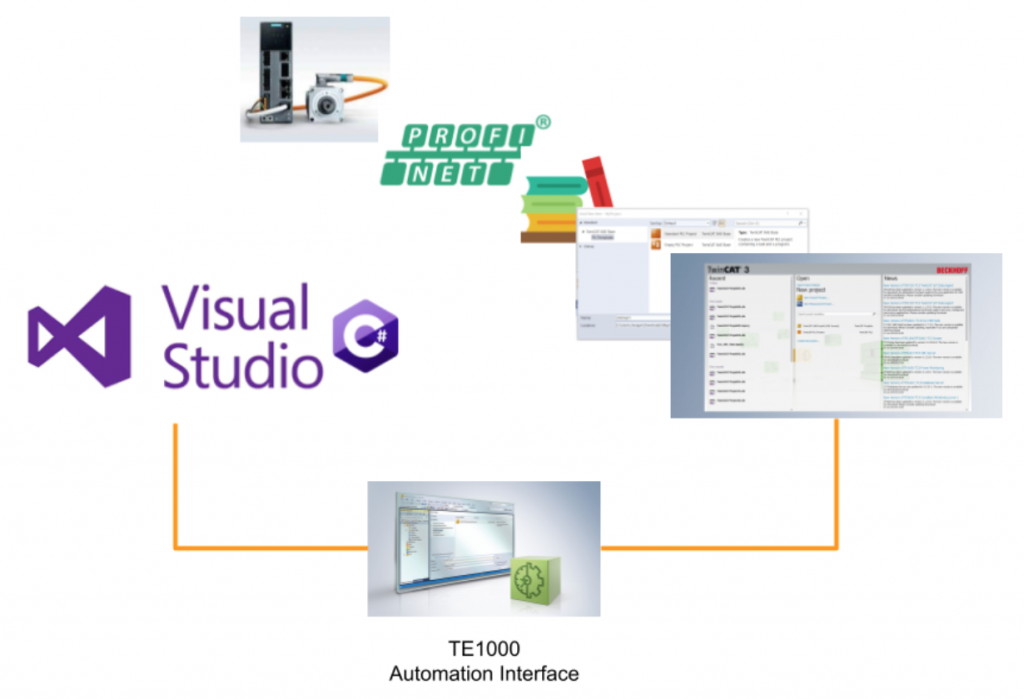
program
using System; using System.IO; using TCatSysManagerLib; namespace ConsoleApp2 { class Program { static string getTheCurrentDataTime() { DateTime dt = DateTime.Now; return dt.ToString(“yyyy/MM/dd HH:mm:ss”); } static void Main(string[] args) { //Init String MyStandardPLCProjectTemplates = “Standard PLC Template.plcproj”; String PLCName = “MyPLC”; String path = @”C:\Users\chungw\Downloads\Myp”; int S210Telegram30 = 5; int S210Telegram3 = 7; //Creating the Visutal Studio DTE Console.WriteLine(“{0}:Getting VisualStudio DTE ID…”, getTheCurrentDataTime()); Type t = System.Type.GetTypeFromProgID(“VisualStudio.DTE.16.0”); Console.WriteLine(“{0}:VisualStudio DTE ID is read!”, getTheCurrentDataTime()); //Create the Instance Console.WriteLine(“{0}:Creating the Instance..”, getTheCurrentDataTime()); EnvDTE.DTE dte = (EnvDTE.DTE)System.Activator.CreateInstance(t); dte.SuppressUI = false; dte.MainWindow.Visible = true; Console.WriteLine(“{0}:Start to Create Folder..”, getTheCurrentDataTime()); DirectoryInfo di = new DirectoryInfo(path); di.Create(); dte.Solution.Create(path, “MySolution1”); dte.Solution.SaveAs(@”C:\Users\chungw\Downloads\Myp\Solution1.sln”); //Add Solution string template = @”C:\TwinCAT\3.1\Components\Base\PrjTemplate\TwinCAT Project.tsproj”; ITcSysManager sysManager = (ITcSysManager)dte.Solution.AddFromTemplate(template, @”C:\Users\chungw\Downloads\Myp\Solution1″, “MyProject”).Object; //PLC Console.WriteLine(“{0}:TwinCAT is started.”, getTheCurrentDataTime()); ITcSmTreeItem plc = sysManager.LookupTreeItem(“TIPC”); Console.WriteLine(“{0}:Creating PLC…”, getTheCurrentDataTime()); ITcSmTreeItem newp = plc.CreateChild(PLCName, 0, “”, MyStandardPLCProjectTemplates); //Get PLC Project ITcSmTreeItem plcProject = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project”); ITcPlcIECProject importExport = (ITcPlcIECProject)plcProject; //ITcSmTreeItem GVL = plcProject.CreateChild(“GVL_1”, 615, null, null); importExport.PlcOpenImport(@”C:\Users\chungw\Downloads\Myp\GVL_1.xml”, (int)PLCIMPORTOPTIONS.PLCIMPORTOPTIONS_NONE); //Reference Library ITcSmTreeItem references = sysManager.LookupTreeItem(“TIPC^MyPLC^MyPLC Project^References”); ITcPlcLibraryManager libManager = (ITcPlcLibraryManager)references; libManager.AddLibrary(“Tc2_MC2”, “*”, “Beckhoff Automation GmbH”); libManager.AddLibrary(“Tc2_MC2_Drive”, “*”, “Beckhoff Automation GmbH”); //Create the Profinet IO Controllers Console.WriteLine(“{0}:Createing the Profinet IO Controller..”, getTheCurrentDataTime()); ITcSmTreeItem io = sysManager.LookupTreeItem(“TIID”); ITcSmTreeItem ProfinetController = io.CreateChild(“PNIO Controller”, 113, null, null); ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><IP>#x0103a8c0</IP></IpSettings></DevicePnControllerDef></TreeItem>”); ProfinetController.ConsumeXml(“<TreeItem><DevicePnControllerDef><IpSettings><Subnet>#x00ffffff</Subnet></IpSettings></DevicePnControllerDef></TreeItem>”); //Create S210 Console.WriteLine(“{0}:Createing the Profinet IO Devices of S210..”, getTheCurrentDataTime()); ITcSmTreeItem S210_1 = ProfinetController.CreateChild(“PNDevices_1_S210″, 97, null, @”C:\TwinCAT\3.1\Config\Io\Profinet\GSDML-V2.25-Siemens-Sinamics_S210-20220506.xml#0x0002020C”); //Change the Box Name Console.WriteLine(“{0}:Changing the Box Name..”, getTheCurrentDataTime()); S210_1.ConsumeXml(“<TreeItem><ItemName>box1234</ItemName></TreeItem>”); //Change the IP Console.WriteLine(“{0}:Changing the IP..”, getTheCurrentDataTime()); S210_1.ConsumeXml(“<TreeItem><PnIoBoxDef><IpSettings><IP>#x0a03a8c0</IP></IpSettings></PnIoBoxDef></TreeItem>”); S210_1.ConsumeXml(“<TreeItem><PnIoBoxDef><IpSettings><Gateway>#x0103a8c0</Gateway></IpSettings></PnIoBoxDef></TreeItem>”); ITcSmTreeItem S210_1API = S210_1.get_Child(1); ITcSmTreeItem sub = S210_1API.get_Child(2); Console.WriteLine(“{0}:inserting the Telegram..”, getTheCurrentDataTime()); ITcSmTreeItem sub2 = sub.CreateChild(“ProfiSAFE_Telegram30”, S210Telegram30, null, null); ITcSmTreeItem sub3 = sub.CreateChild(“StandardTelegram3”, S210Telegram3, null, null); } } } |
Result
A Profinet Device that is called box1234 has been added under Done!PNIO Controller.
And Telegram30, Standard3 are also installed.
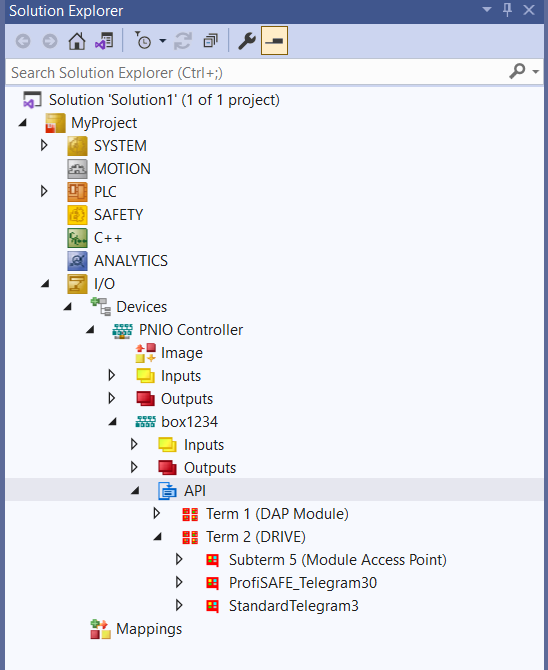
In the example I added S210 under the Profinet Controller by using the CreateChild Method.
//Create S210 Console.WriteLine(“{0}:Createing the Profinet IO Devices of S210..”, getTheCurrentDataTime()); ITcSmTreeItem S210_1 = ProfinetController.CreateChild(“PNDevices_1_S210”, 97, null, @”C:\TwinCAT\3.1\Config\Io\Profinet\GSDML-V2.25-Siemens-Sinamics_S210-20220506.xml#0x0002020C”); |
Path in GSDML for parameter
GSDML-V2.25-Siemens-Sinamics_S210-20220506.xml#0x0002020C.
#0x0002020C will specify the corresponding Profinet Devices version.
and #0x0002020C is V5.1.
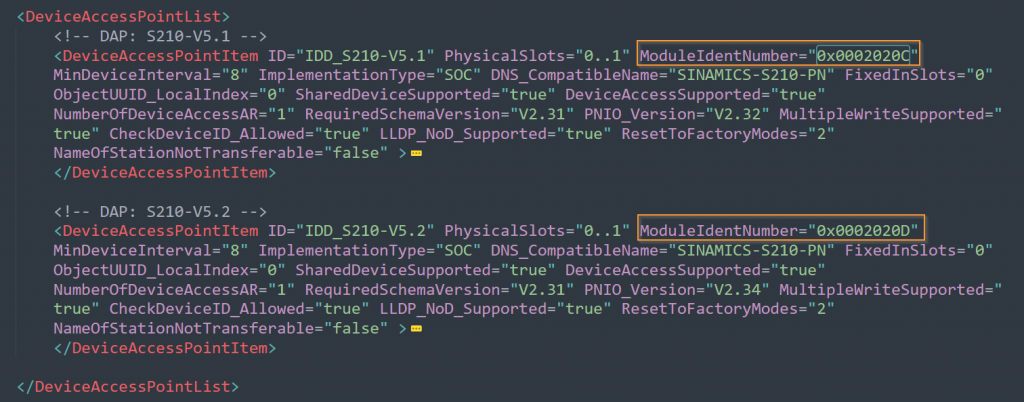
This Child is an API Object for Profinet devices.
ITcSmTreeItem S210_1API = S210_1.get_Child(1); |
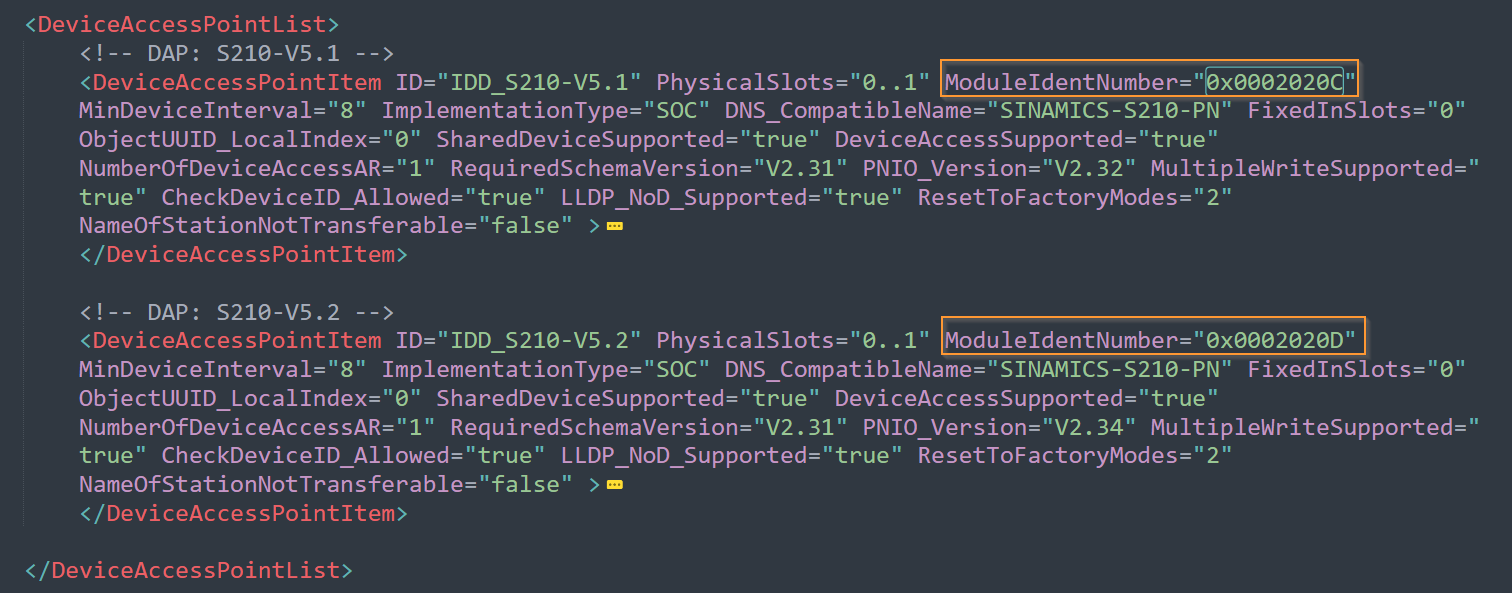
Furthermore, this Child will be Term2 (Drive).
ITcSmTreeItem sub = S210_1API.get_Child(2); |
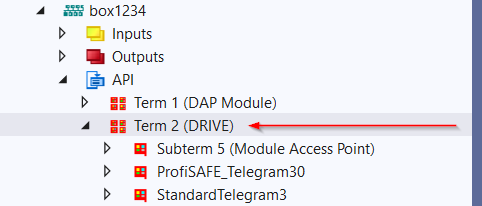
Finally ProfiSAFE Telegram30 and Standard Telegram3 are added in our configuration.
ITcSmTreeItem sub2 = sub.CreateChild(“ProfiSAFE_Telegram30”, S210Telegram30, null, null); ITcSmTreeItem sub3 = sub.CreateChild(“StandardTelegram3”, S210Telegram3, null, null); |
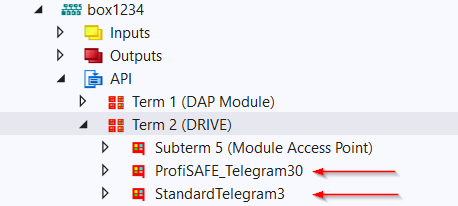