This article I will use Omron’s Sysmac Library for MQTT communication, a combination of PLCNEXT and Podman’s Container technology on the Broker side, but a regular Raspberry Pi will also work.
Let’s start!
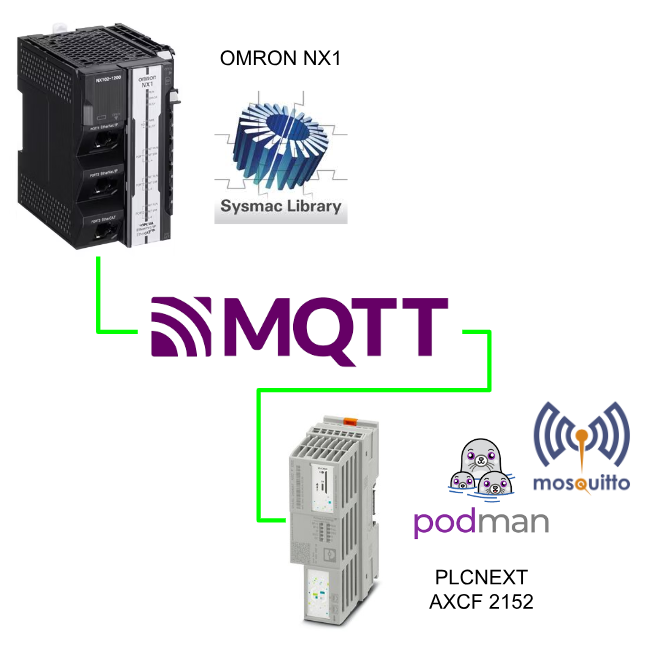
Reference Link
Build a Broker
MQTT Program With Other CPU
MQTT Library?
The MQTT Communications Library is a library that allows OMRON NX CPU to use the software features for Pub/Sub type message exchange with MQTT Broker.
This library supports version 3.1.1 of the MQTT protocol.
Using the MQTT Communications Library, such functionality can be implemented:
- Connect with MQTT Broker
- As a Subscriber, receive information from Publishers in the network.
- As a Publisher, sends information to a Subscriber in the network.
- Communicate with MQTT Broker and check response time
Install
Download the MQTT Communication library from Omron HP and start the EXE.
https://www.fa.omron.co.jp/product/tool/sysmac-library/
Choose language >OK to continue.
Proceed with Next>.
Agree to the license and proceed with Next>.
Proceed with Next>.
Install!
Just a second..
Done!
Method
Next, we will introduce the Function Block and DUT used in this article.
DUT
sConnectionSettings
Name | Data Type | Description |
IpAdr | STRING[201] | Broker’s IP Address |
PortNo | UINT | Broker’s Port Number |
TLSUse | BOOL | 1=TLS is used |
TLSessionName | STRING[17] | TLS session name |
UserName | STRING[256] | Login’s Username |
Password | STRING[256] | Login’s Password |
WillCfg | sWillCfg | Will parameters |
CleanSession | BOOL | 1=Session is cleared. |
sWillCfg
Name | Data Type | Description |
WillTopic | STRING[512] | Will Topic’s name |
WillMsg | STRING[256] | Will Message |
WillRetain | BOOL | 1=Retain the Message |
WillQoS | BYTE | QoS Leve(0,1,2) |
WillFlag | BOOL | 1=Will function is enabled |
MQTTClient
This Function Block creates a connection between the NX CPU and the MQTT Broker, and after the MQTTClient is successfully executed, the INOUT parameter, ClientReference, is set to MQTTPubAryByte, MQTTSubAryByte, MQTTSubString, MQTTPing. MQTTSubString, and MQTTPing Function Block.
Input Variables
Variable | Data type | Description |
Enable | BOOL | True=start to connect |
ClientID | STRING[256] | Identifier for the MQTT Broker to identify |
ConnectionSettings | OmronLib\MQTT_Comm\sConnectionSettings | Parameters to connect to the MQTT Broker |
KeepAlive | UINT | KeepAlive time setting 0-65535 |
Timeout | UINT | Timeout setting(0-20s) |
DiscardMsgTime | UINT | Specifies the maximum time to wait for a received message to be discarded. |
Output Variables
Variable | Data type | Description |
Connected | BOOL | True=Connecting with Broker |
Busy | BOOL | True=Function Block is running |
Error | BOOL | True=Function Block error occurred |
ErrorID | WORD | 0=Function Block terminated normally, others have Function Block errors. |
ErrorIDEx | DWORD | Error Code Extensions |
DiscardMsgNum | UDINT | This is the number of discarded message receptions |
SessionPresent | BOOL | True=Session is present. |
VAR_IN_OUT
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
MQTTPubAryByte
This Function Block sends a PUBLISH message of the message specified in the BYTE array to the MQTT broker.
VAR_IN
Name | Type | Description |
Execute | BOOL | Function Block will execute at startup |
MsgSize | UINT | Size of Publish message in parameter PubMsg (Byte) |
PubSettings | sPubFlags | Retain and QoS settings when publishing |
Topic | STRING[512] | Topic name to publish |
TimeOut | UINT | Timeout Settings |
VAR_OUT
Name | Type | Description |
Done | BOOL | True=Connecting with Broker |
Busy | BOOL | True=Function Block is running |
Error | BOOL | True=Function Block error occurred |
ErrorID | WORD | 0=Function Block terminated normally, others have Function Block errors. |
ErrorIDEx | DWORD | Error Code Extensions |
VAR_IN_OUT
Name | Type | Description |
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
PubMsg | ARRAY[*] OF BYTE | Message to be sent to the Broker |
MsgSize | UINT | Size of Publish message in parameter PubMsg (Byte) |
PacketID | UINT | If a number other than 0 is specified at the start of execution, the message is sent as a retransmission message for the specified packet ID. |
MsgType | USINT | The parameter is accessed if the QoS level is 1 or 2 and the PacketID is not 0. 0=PUBLISH, 1=PUBREL |
MQTTPubString
This Function Block sends a PUBLISH message of the message specified by the string to the MQTT broker.
VAR_IN
Name | Type | Description |
Execute | BOOL | Function Block will execute at startup |
PubSettings | sPubFlags | Retain and QoS settings when publishing |
Topic | STRING[512] | Topic name to publish |
TimeOut | UINT | Timeout Settings |
VAR_OUT
Name | Type | Description |
Done | BOOL | True=Connecting with Broker |
Busy | BOOL | True=Function Block is running |
Error | BOOL | True=Function Block error occurred |
ErrorID | WORD | 0=Function Block terminated normally, others have Function Block errors. |
ErrorIDEx | DWORD | Error Code Extensions |
VAR_IN_OUT
Name | Type | Description |
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
PubMsg | ARRAY[*] OF BYTE | Message to be sent to the Broker |
MsgSize | UINT | Size of Publish message in parameter PubMsg (Byte) |
PacketID | UINT | If a number other than 0 is specified at the start of execution, the message is sent as a retransmission message for the specified packet ID. |
MsgType | USINT | The parameter is accessed if the QoS level is 1 or 2 and the PacketID is not 0. 0=PUBLISH, 1=PUBREL |
MQTTSubAryByte
This Function Block starts a subscription to the Broker with the input parameter Enable=True and the receiving the message as a byte array.
VAR_IN
Name | Type | Description |
Enable | BOOL | 1=Star to Subscribe |
Topic | STRING[512] | The Subscribe’s topic |
SubQoS | BYTE | Qos Setting |
TimeOut | UINT | Timeout Settings |
VAR_OUT
Name | Type | Description |
Subscribed | BOOL | 1=Topic is Subscribed |
Status | SINT | Scbscribe status.0:Execution is stopped1:Requesting Subscribe2:Subscribe is in progress3:Stopping Subscribe4:Waiting for Broker to reconnect |
Received | BOOL | 1=Message received |
MsgSize | UINT | Data size received (Byte) |
RcvTopic | STRING[512] | received Topic |
Error | BOOL | True=Function Block error occurred |
ErrorID | WORD | 0=Function Block terminated normally, others have Function Block errors. |
ErrorIDEx | DWORD | Error Code Extensions |
VAR_IN_OUT
Name | Type | Description |
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
RcvMsg | ARRAY[*] OF BYTE | Received data is stored in |
MQTTSubString
The Function Block here initiates a Subscribe to the Broker with the input parameter Enable=True and receiving the message as a string.
VAR_IN
Name | Type | Description |
Enable | BOOL | 1=Star to Subscribe |
Topic | STRING[512] | The Subscribe’s topic |
SubQoS | BYTE | Qos Setting |
TimeOut | UINT | Timeout Settings |
VAR_OUT
Name | Type | Description |
Subscribed | BOOL | 1=Topic is Subscribed |
Status | SINT | Scbscribe status.0:Execution is stopped1:Requesting Subscribe2:Subscribe is in progress3:Stopping Subscribe4:Waiting for Broker to reconnect |
Received | BOOL | 1=Message received |
RcvTopic | STRING[512] | Data size received (Byte) |
Error | BOOL | received Topic |
ErrorID | WORD | True=Function Block error occurred |
ErrorIDEx | DWORD | 0=Function Block terminated normally, others have Function Block errors. |
VAR_IN_OUT
Name | Type | Description |
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
RcvMsg | ARRAY[*] OF BYTE | Received data is stored in |
MQTTPing
This Function Block can send ping messages to the MQTT Broker and measure the response time.
VAR_IN
Name | Type | Description |
Enable | BOOL | 1=Start to ping |
TimeOut | UINT | Timeout setting |
VAR_OUT
Name | Type | Description |
Done | BOOL | 1=FB execution success |
Busy | BOOL | 1=FB is busy |
ElapseTime | UINT | Time between sending a PINGREQ packet and receiving a PINGRESP packet |
Error | BOOL | True=Function Block error occurred |
ErrorID | WORD | 0=Function Block terminated normally, others have Function Block errors. |
ErrorIDEx | DWORD | Error Code Extensions |
VAR_IN_OUT
Name | Type | Description |
ClientReference | sClientReference | Data to be shared among MQTT FBs. |
Implementation
Import the library
Start Sysmac Studio and import the library into your project.
Go to Project>Library>Show References.
Click the + button.
Select the library you have just downloaded and open it.
It is OK to ignore.
MQTT Library has been imported.
Configuration
Open Controller Setup>Built-in EtherNet/IP Settings and set the IP of the port connected to the MQTT Broker from the TCP/IP Setting tab.
Program
DUT
DUT_MQTT_Basic
DUT_MQTT_Client
DUT_MQTT_Sub
DUT_MQTT_Ping
DUT_MQTT_Pub
VAR
Variables Name | Type | Descritpion |
bDummy | BOOL | |
bAlwaysON | BOOL | |
pubFlags | OmronLib\MQTT_Comm\sPubFlags | Configuration to Publish Message to Broker |
rcvMsg | ARRAY [0..999] OF BYTE | Destination of the Byte array received in the Broker |
RcvTopic | STRING[512] | Topic name received in the Broker |
SubMessage | STRING[1986] | String data received in the Broker is stored at |
MyString | STRING[255] | |
pubMsg | ARRAY[0..999] OF BYTE | Byte array data to be published to the Broker |
StringToAry_DataConverted | UINTを | Data Conversion Buffer |
MQTTPubString | OmronLib\MQTT_Comm\MQTTPubString | FB Instance of Publish string data to Broker |
PubMessage | STRING[1986] | Destination of string data to be published to the Broker |
MQTTClient | OmronLib\MQTT_Comm\MQTTClient | FB Instance to connect with Broker |
bMQTTConnect | BOOL | 1=Connect with MQTT Broker |
Client | DUT_MQTT_Client | FB Parameter Variables |
MQTTSubAryByte | OmronLib\MQTT_Comm\MQTTSubAryByte | BrokerにMessageをSubscribe FB Instance |
SubAryByte | DUT_MQTT_Sub | FB Parameter Variables |
MQTTPing | OmronLib\MQTT_Comm\MQTTPing | FB Instance to ping the Broker |
MQTTSubString | OmronLib\MQTT_Comm\MQTTSubString | BrokerにMessageをSubscribe FB Instance |
Ping | DUT_MQTT_Ping | FB Parameter Variables |
SubString | DUT_MQTT_Sub | FB Parameter Variables |
PubAryByte | DUT_MQTT_Pub | FB Parameter Variables |
MQTTPubAryByte | OmronLib\MQTT_Comm\MQTTPubAryByte | Destination of Byte array data to be published to the Broker |
pubString | DUT_MQTT_Pub | FB Parameter Variables |
Result_SubAryByte | DINT | |
Result_SubString | DINT |
Rung0
Summarize Rung Comment.
Rung1
Set parameters to connect to the MQTT Broker.
Rung2
Set TLS parameters to connect to the MQTT Broker.
Rung3
Configure the WillCfg settings.
Rung4
Enable the CleanSession option.
Rung5
Configure the Qos and Retain settings when the Omron NX CPU publishes a message.
Rung6
bMQTTConnect is set to True when the Ethernet Port of the OMRON NX CPU is normal and the MQTT Function is not running or has not generated any errors. The bMQTTConnect is a parameter of the MQTTClient Function.
Rung7
Summarize the Rung Comment with a Dummy circuit.
Rung8
Call the MQTTClient Function Block to connect to the MQTT Broker.
Rung9
If the MQTT Client connects to the Broker OK and no error occurs, it will automatically subscribe the Topic to the Broker.
Rung10
Subscribe Topic PLCNEXT/Node/Data/Node1 from Broker by calling MQTTSubAryByte Function Block.。
Rung11
When a new Message is received, the data is converted from a Byte array to a string using the AryToString function, and then the string is reconverted to DINT.
Rung12
Check the communication status between NX CPU and Broker using MQTTPing Function Block.
Rung13
Subscribe Topic PLCNEXT/Node/Data/Node1 from Broker by calling MQTTSubString Function Block.
Rung14
When a new Message is received, the data is converted from string to DINT using the AryToString function.。
Rung15
Writes the Message value to be published when the NX CPU is connected to the Broker.
Rung16
Call MQTTPubAryByte Function Block to Publish Message to Broker.
Rung17
Reset the trigger device once the Message is published.
Rung18
Writes the Message value to be published when the NX CPU is connected to the Broker.
Rung19
Call MQTTPubString Function Block to Publish Message to Broker.
Rung20
Reset the trigger device once the Message is published.
Result
Done!Client.Connected is now Ture and the NX CPU has connected to the Broker.
Subscribe
Omron NX CPU subscribed “PLCNEXT/Node/Data/Node1” to the MQTT Broker.
Now, let’s try to Publish the data on the PLCNEXT side.
SubAryByte.MsgSize=5, i.e. 5Bytes of data received.
You can then see the Topic received from RevTopic.
Data 12345 received.
You can check the Topic received from RevTopic in the MQTTSubString Function Block.
Likewise, data 12345 was received.
Publish
Now we will trigger the Execute Flag of MQTTPubAryBytePublish and send data from the Omron NX CPU to the Broker in PLCNEXT.。
Done!PLC NEXT Broker received 7891.
Next, MQTTPubString’s Execute is triggered and the string data is sent to the PLC NEXT Broker.
Done!PLC NEXT Broker received 8922 numbers.